编写一个学生和教师显示程序,用带参数的构造函数赋初值
时间: 2023-11-28 15:47:57 浏览: 79
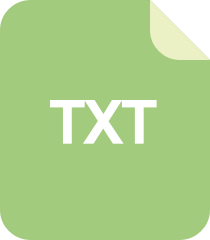
用C++编写一个教师和学生数据的输入和显示程序
以下是一个基于C++的示例程序,用于展示如何使用带参数的构造函数来初始化学生和教师对象,并显示它们的信息:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
int age;
public:
Person(string n, int a) {
name = n;
age = a;
}
virtual void display() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
}
};
class Student : public Person {
private:
int grade;
public:
Student(string n, int a, int g) : Person(n, a) {
grade = g;
}
void display() {
cout << "Student Information: " << endl;
Person::display();
cout << "Grade: " << grade << endl;
}
};
class Teacher : public Person {
private:
string department;
public:
Teacher(string n, int a, string d) : Person(n, a) {
department = d;
}
void display() {
cout << "Teacher Information: " << endl;
Person::display();
cout << "Department: " << department << endl;
}
};
int main() {
Student s("Tom", 18, 12);
s.display();
Teacher t("Mary", 35, "Math");
t.display();
return 0;
}
```
在上面的示例程序中,我们定义了一个基类 Person,以及两个派生类 Student 和 Teacher,它们都继承自 Person。在 Person 中,我们定义了一个带参数的构造函数,用于初始化 name 和 age 两个成员变量。在 Student 和 Teacher 中,我们也定义了带参数的构造函数,并在初始化列表中调用了基类的构造函数来初始化基类的成员变量。在主函数中,我们创建了一个 Student 对象和一个 Teacher 对象,并调用它们的 display 函数来显示它们的信息。
阅读全文
相关推荐
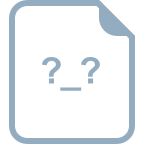




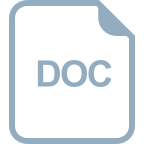











