用遗传算法计算最大值c++
时间: 2023-07-24 20:26:00 浏览: 98
好的,我可以为您提供一个遗传算法计算最大值的C++示例代码,以下是一个简单的遗传算法实现:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <cmath>
using namespace std;
// 定义常量
const int POP_SIZE = 10; // 种群大小
const int MAX_GEN = 100; // 最大迭代次数
const double MUTATION_RATE = 0.1; // 突变概率
const double CROSSOVER_RATE = 0.6; // 交叉概率
// 定义染色体类
class Chromosome{
public:
Chromosome(); // 构造函数
double fitness(); // 适应度函数
void mutate(); // 突变操作
Chromosome crossover(Chromosome x); // 交叉操作
double getGene(); // 获取基因
void setGene(double x); // 设置基因
private:
double gene; // 基因
};
// 构造函数
Chromosome::Chromosome(){
gene = (double)rand() / RAND_MAX * 10; // 随机初始化基因
}
// 计算适应度函数
double Chromosome::fitness(){
return pow(gene, 2);
}
// 突变操作
void Chromosome::mutate(){
if((double)rand() / RAND_MAX < MUTATION_RATE){
gene = (double)rand() / RAND_MAX * 10;
}
}
// 交叉操作
Chromosome Chromosome::crossover(Chromosome x){
Chromosome child;
if((double)rand() / RAND_MAX < CROSSOVER_RATE){
child.setGene((gene + x.getGene()) / 2);
}else{
child.setGene(gene);
}
return child;
}
// 获取基因
double Chromosome::getGene(){
return gene;
}
// 设置基因
void Chromosome::setGene(double x){
gene = x;
}
// 定义种群类
class Population{
public:
Population(); // 构造函数
void evolve(); // 进化函数
Chromosome getBest(); // 获取最优解
void print(); // 打印种群信息
private:
Chromosome pop[POP_SIZE]; // 种群
};
// 构造函数
Population::Population(){
srand((unsigned)time(NULL));
}
// 进化函数
void Population::evolve(){
for(int i = 0; i < MAX_GEN; i++){
// 计算种群中每个染色体的适应度
double total_fitness = 0;
double best_fitness = 0;
int best_index = 0;
for(int j = 0; j < POP_SIZE; j++){
double f = pop[j].fitness();
if(f > best_fitness){
best_fitness = f;
best_index = j;
}
total_fitness += f;
}
// 计算每个染色体的选择概率
double p[POP_SIZE];
for(int j = 0; j < POP_SIZE; j++){
p[j] = pop[j].fitness() / total_fitness;
}
// 生成新的种群
Chromosome new_pop[POP_SIZE];
for(int j = 0; j < POP_SIZE; j++){
// 选择操作
double r = (double)rand() / RAND_MAX;
double sum = 0;
int k = 0;
while(sum < r){
sum += p[k];
k++;
}
k--;
// 交叉操作
Chromosome child;
child = pop[k].crossover(pop[rand() % POP_SIZE]);
// 突变操作
child.mutate();
new_pop[j] = child;
}
// 替换旧种群
for(int j = 0; j < POP_SIZE; j++){
pop[j] = new_pop[j];
}
}
}
// 获取最优解
Chromosome Population::getBest(){
double best_fitness = 0;
int best_index = 0;
for(int i = 0; i < POP_SIZE; i++){
double f = pop[i].fitness();
if(f > best_fitness){
best_fitness = f;
best_index = i;
}
}
return pop[best_index];
}
// 打印种群信息
void Population::print(){
for(int i = 0; i < POP_SIZE; i++){
cout << "Chromosome " << i << ": gene = " << pop[i].getGene() << ", fitness = " << pop[i].fitness() << endl;
}
}
// 主函数
int main(){
Population pop;
pop.print();
pop.evolve();
Chromosome best = pop.getBest();
cout << "Best solution: gene = " << best.getGene() << ", fitness = " << best.fitness() << endl;
return 0;
}
```
这段代码实现的是一个简单的遗传算法,其中`Chromosome`类表示染色体,`Population`类表示种群。在`Population::evolve()`函数中,遗传算法的主要步骤包括:
1. 计算种群中每个染色体的适应度;
2. 计算每个染色体的选择概率;
3. 生成新的种群,包括选择、交叉、突变操作;
4. 替换旧种群;
5. 重复上述步骤直到达到最大迭代次数。
在本例中,适应度函数为基因的平方,所以最大值即为基因为10的染色体。
阅读全文
相关推荐












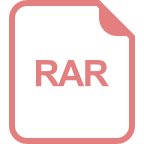

