遗传算法求函数最大值,并给出C++示例
时间: 2024-05-05 12:10:20 浏览: 125
遗传算法是一种基于生物进化原理的优化算法,可以用于求解函数最大值问题。其基本思路是通过模拟自然选择、交叉和变异等生物进化过程,从而逐步优化得到最优解。
下面是一个简单的遗传算法的C++示例,用于求解函数f(x) = x^2在区间[-10, 10]上的最大值:
```c++
#include <iostream>
#include <cmath>
#include <random>
#include <algorithm>
#include <vector>
using namespace std;
// 目标函数
double f(double x) {
return x * x;
}
// 遗传算法参数
const int POPULATION_SIZE = 100; // 种群大小
const int MAX_GENERATION = 100; // 最大迭代次数
const double CROSSOVER_RATE = 0.8; // 交叉概率
const double MUTATION_RATE = 0.01; // 变异概率
// 个体结构体
struct Individual {
double x; // 自变量
double fitness; // 适应度
// 构造函数
Individual(double x) {
this->x = x;
fitness = f(x);
}
// 重载小于运算符
bool operator<(const Individual& other) const {
return fitness > other.fitness;
}
};
// 随机数生成器
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(-10, 10);
// 初始化种群
vector<Individual> init_population() {
vector<Individual> population;
for (int i = 0; i < POPULATION_SIZE; i++) {
double x = dis(gen);
population.emplace_back(x);
}
return population;
}
// 计算适应度
void evaluate(vector<Individual>& population) {
for (auto& ind : population) {
ind.fitness = f(ind.x);
}
}
// 选择操作
Individual select(const vector<Individual>& population) {
uniform_int_distribution<> dis(0, POPULATION_SIZE - 1);
int index1 = dis(gen);
int index2 = dis(gen);
return population[index1].fitness > population[index2].fitness ?
population[index1] : population[index2];
}
// 交叉操作
Individual crossover(const Individual& parent1, const Individual& parent2) {
uniform_real_distribution<> dis(0, 1);
if (dis(gen) > CROSSOVER_RATE) {
return parent1;
}
double x = (parent1.x + parent2.x) / 2;
return Individual(x);
}
// 变异操作
void mutate(Individual& ind) {
uniform_real_distribution<> dis(-1, 1);
if (dis(gen) > MUTATION_RATE) {
return;
}
ind.x += dis(gen);
}
// 遗传算法主函数
double genetic_algorithm() {
vector<Individual> population = init_population();
evaluate(population);
sort(population.begin(), population.end());
for (int i = 0; i < MAX_GENERATION; i++) {
vector<Individual> new_population;
while (new_population.size() < POPULATION_SIZE) {
Individual parent1 = select(population);
Individual parent2 = select(population);
Individual child = crossover(parent1, parent2);
mutate(child);
new_population.emplace_back(child);
}
evaluate(new_population);
sort(new_population.begin(), new_population.end());
population = new_population;
}
return population.front().x;
}
int main() {
double x_max = genetic_algorithm();
cout << "x_max = " << x_max << ", f(x_max) = " << f(x_max) << endl;
return 0;
}
```
该示例中,我们首先定义了目标函数f(x),然后定义了遗传算法的一些参数和个体结构体。在初始化种群时,我们使用了C++11中的emplace_back函数,可以避免不必要的拷贝操作,提高程序效率。在遗传算法的主函数中,我们首先进行了种群初始化和适应度计算,然后进行了迭代过程。在每一次迭代中,我们使用了轮盘赌选择和简单的算术交叉和随机变异操作。最终,我们输出了求得的最大值x_max及其对应的函数值f(x_max)。
需要注意的是,遗传算法只能求解单峰函数的最大值,对于多峰函数或者具有约束条件的问题,可能需要使用其他的优化算法。
阅读全文
相关推荐
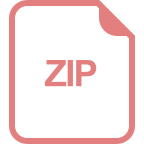
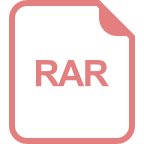
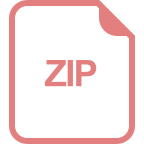















