GeoTools wkt 转 geojson,maven里的依赖jar如何配置
时间: 2024-02-26 18:57:14 浏览: 194
将以下依赖项添加到您的Maven项目中的pom.xml文件中:
```xml
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geojsondatastore</artifactId>
<version>23.5</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-epsg-hsql</artifactId>
<version>23.5</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-referencing</artifactId>
<version>23.5</version>
</dependency>
<dependency>
<groupId>org.locationtech.jts</groupId>
<artifactId>jts-core</artifactId>
<version>1.16.1</version>
</dependency>
```
这些依赖项将提供用于将WKT转换为GeoJSON的类和方法。请注意,这些依赖项的版本号可能会随时间而变化,您应该选择与您正在使用的GeoTools版本兼容的版本。
相关问题
GeoTools wkt 转 geojson
可以使用GeoTools库来实现WKT转换为GeoJSON。以下是一个Java代码示例:
```java
import org.geotools.geojson.geom.GeometryJSON;
import org.locationtech.jts.geom.Geometry;
import org.locationtech.jts.io.ParseException;
import org.locationtech.jts.io.WKTReader;
import java.io.IOException;
import java.io.StringWriter;
public class WktToGeojsonConverter {
public static String convert(String wkt) throws ParseException, IOException {
WKTReader reader = new WKTReader();
Geometry geometry = reader.read(wkt);
StringWriter writer = new StringWriter();
GeometryJSON gjson = new GeometryJSON();
gjson.write(geometry, writer);
return writer.toString();
}
}
```
你可以将WKT作为参数传递给convert()方法,然后它将返回一个GeoJSON字符串。
geotools解析geojson
GeoTools是一个开源的Java工具包,可用于处理地理空间数据。GeoTools提供了对GeoJSON格式的支持,可以使用GeoTools解析GeoJSON数据。
以下是一个简单的示例代码,展示了如何使用GeoTools解析GeoJSON数据:
```java
File geojsonFile = new File("path/to/geojson/file"); // GeoJSON文件路径
// 创建GeoJSON文件读取器
GeoJSONDataStoreFactory dataStoreFactory = new GeoJSONDataStoreFactory();
Map<String, Object> params = new HashMap<>();
params.put(GeoJSONDataStoreFactory.URLP.key, geojsonFile.toURI().toURL());
GeoJSONDataStore dataStore = (GeoJSONDataStore) dataStoreFactory.createDataStore(params);
// 获取GeoJSON数据源中的FeatureCollection
SimpleFeatureSource featureSource = dataStore.getFeatureSource(dataStore.getTypeNames()[0]);
SimpleFeatureCollection featureCollection = featureSource.getFeatures();
// 遍历FeatureCollection
try (SimpleFeatureIterator featureIterator = featureCollection.features()) {
while (featureIterator.hasNext()) {
SimpleFeature feature = featureIterator.next();
Geometry geometry = (Geometry) feature.getDefaultGeometry(); // 获取几何对象
// 对几何对象进行操作
// ...
}
}
```
上述代码首先创建了一个GeoJSON文件读取器,然后使用该读取器获取GeoJSON数据源中的FeatureCollection。接着遍历FeatureCollection,对其中的每个Feature进行操作。
需要注意的是,使用GeoTools解析GeoJSON数据时,需要先将GeoJSON文件转换为GeoJSON数据源,然后再从数据源中获取FeatureCollection。GeoTools支持的数据源类型包括文件、URL、InputStream等。
阅读全文
相关推荐
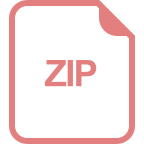
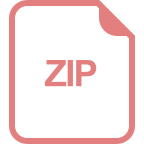
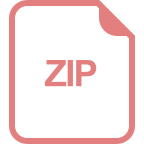
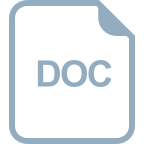
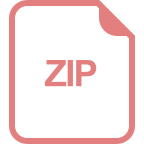










