设有一个点类Point的定义如下: Point { public: Point() {x = 0; y = 0; } Point(double xv,double yv) {x = xv;y = yv;} Point(Point& pt) { x = pt.x; y = pt.y; } double getx() { return x; } double gety() { return y; } double Area() { return 0; } void Show() { cout<<"x="<<x<<' '<<"y="<<y<<endl; } private: double x,y; }; 编写程序,以点point类为基类,派生出矩形类Rectangle和圆类Circle。矩形由左上角的顶点和长、宽定义。圆由圆心和半径定义。派生类Circle中新增的成员函数position(Point &pt)用于判断任一坐标点是在圆内、还是在圆的边缘上,还是在圆外。 [附
时间: 2023-06-20 07:10:31 浏览: 115
代码实现]
```
#include <iostream>
#include <cmath>
using namespace std;
class Point {
public:
Point() { x = 0; y = 0; }
Point(double xv, double yv) { x = xv; y = yv; }
Point(Point& pt) { x = pt.x; y = pt.y; }
double getx() { return x; }
double gety() { return y; }
double Area() { return 0; }
void Show() { cout << "x=" << x << ' ' << "y=" << y << endl; }
private:
double x, y;
};
class Rectangle : public Point {
public:
Rectangle() { x = 0; y = 0; width = 0; height = 0; }
Rectangle(double xv, double yv, double w, double h) { x = xv; y = yv; width = w; height = h; }
double getWidth() { return width; }
double getHeight() { return height; }
double Area() { return width * height; }
private:
double width, height;
};
class Circle : public Point {
public:
Circle() { x = 0; y = 0; radius = 0; }
Circle(double xv, double yv, double r) { x = xv; y = yv; radius = r; }
double getRadius() { return radius; }
double Area() { return M_PI * radius * radius; }
int position(Point& pt) {
double distance = sqrt((pt.getx() - x) * (pt.getx() - x) + (pt.gety() - y) * (pt.gety() - y));
if (distance < radius) {
return 1; // 在圆内
}
else if (distance == radius) {
return 0; // 在圆的边缘上
}
else {
return -1; // 在圆外
}
}
private:
double radius;
};
int main() {
Rectangle rect(2, 2, 3, 4);
Circle cir(5, 5, 2);
Point pt1(3, 3);
Point pt2(6, 6);
Point pt3(7, 7);
cout << "Rectangle Area: " << rect.Area() << endl;
cout << "Circle Area: " << cir.Area() << endl;
cout << "Point1 position: " << cir.position(pt1) << endl;
cout << "Point2 position: " << cir.position(pt2) << endl;
cout << "Point3 position: " << cir.position(pt3) << endl;
return 0;
}
```
上面的代码中,我们定义了一个 `Point` 类作为基类,然后通过继承的方式派生出了 `Rectangle` 类和 `Circle` 类。
其中,`Rectangle` 类表示矩形,包含左上角的顶点和长、宽两个属性。`Circle` 类表示圆,包含圆心和半径两个属性。
在 `Circle` 类中,我们新增了一个成员函数 `position`,用于判断任意一个坐标点是在圆内、还是在圆的边缘上,还是在圆外。该函数的实现是通过计算该点与圆心之间的距离来进行判断的。
在 `main` 函数中,我们分别创建了一个矩形对象和一个圆对象,并演示了它们的面积计算以及圆内、边缘、外部点的位置判断。
相关推荐
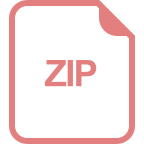
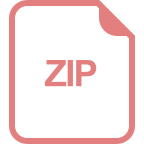
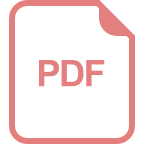














