sgp30气体基于51测量气体的程序
时间: 2023-07-16 11:17:31 浏览: 127
以下是一个基于51单片机的SGP30气体传感器测量程序示例,你可以参考一下:
```c
#include <reg52.h>
#include <stdio.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit SDA = P2^0; // I2C总线数据线
sbit SCL = P2^1; // I2C总线时钟线
// SGP30 I2C地址
#define SGP30_ADDR 0x58
// SGP30命令码
#define SGP30_CMD_IAQ_INIT 0x2003
#define SGP30_CMD_IAQ_MEASURE 0x2008
#define SGP30_CMD_GET_BASELINE 0x2015
#define SGP30_CMD_SET_BASELINE 0x201E
#define SGP30_CMD_MEASURE_RAW_SIGNAL 0x2050
#define SGP30_CMD_MEASURE_TVOC_INCEPTANCE 0x20B7
#define SGP30_CMD_MEASURE_H2_SIGNAL 0x209D
// SGP30的CRC校验多项式
#define CRC_POLYNOMIAL 0x31
// SGP30读取到的数据结构体
typedef struct {
uchar data[6];
uchar crc;
} Sgp30Data;
// SGP30传感器初始化函数
void Sgp30Init()
{
// 发送IAQ初始化命令
uchar buf[2] = {SGP30_CMD_IAQ_INIT >> 8, SGP30_CMD_IAQ_INIT & 0xFF};
I2cStart();
I2cWriteByte(SGP30_ADDR << 1);
I2cWriteByte(buf[0]);
I2cWriteByte(buf[1]);
I2cStop();
}
// 从SGP30读取数据
uchar Sgp30ReadData(Sgp30Data *data)
{
uchar i, crc = 0;
I2cStart();
I2cWriteByte((SGP30_ADDR << 1) | 0x01);
for (i = 0; i < 6; i++) {
data->data[i] = I2cReadByte();
crc = Sgp30Crc(data->data[i], crc);
}
data->crc = I2cReadByte();
I2cStop();
return crc;
}
// 从SGP30读取一个16位的字
uint Sgp30ReadWord()
{
Sgp30Data data;
uchar crc;
crc = Sgp30ReadData(&data);
if (crc != data.crc) {
return 0xFFFF;
}
return (data.data[0] << 8) | data.data[1];
}
// 计算SGP30的CRC校验值
uchar Sgp30Crc(uchar data, uchar crc)
{
uchar i;
crc ^= data;
for (i = 8; i > 0; i--) {
if (crc & 0x80) {
crc = (crc << 1) ^ CRC_POLYNOMIAL;
} else {
crc <<= 1;
}
}
return crc;
}
// I2C总线起始信号
void I2cStart()
{
SDA = 1;
SCL = 1;
_nop_();
SDA = 0;
_nop_();
SCL = 0;
}
// I2C总线停止信号
void I2cStop()
{
SDA = 0;
SCL = 1;
_nop_();
SDA = 1;
_nop_();
}
// 写一个字节到I2C总线
void I2cWriteByte(uchar dat)
{
uchar i;
for (i = 0; i < 8; i++) {
SDA = (dat & 0x80) ? 1 : 0;
_nop_();
SCL = 1;
_nop_();
SCL = 0;
dat <<= 1;
}
SDA = 1;
_nop_();
SCL = 1;
_nop_();
SCL = 0;
}
// 从I2C总线读一个字节
uchar I2cReadByte()
{
uchar i, dat = 0;
SDA = 1;
for (i = 0; i < 8; i++) {
SCL = 1;
_nop_();
dat <<= 1;
dat |= SDA;
SCL = 0;
_nop_();
}
return dat;
}
void main()
{
uint co2, tvoc;
Sgp30Init();
while (1) {
// 发送IAQ测量命令
uchar buf[2] = {SGP30_CMD_IAQ_MEASURE >> 8, SGP30_CMD_IAQ_MEASURE & 0xFF};
I2cStart();
I2cWriteByte(SGP30_ADDR << 1);
I2cWriteByte(buf[0]);
I2cWriteByte(buf[1]);
I2cStop();
// 等待数据准备好
delay_ms(10);
// 读取CO2和TVOC值
co2 = Sgp30ReadWord();
tvoc = Sgp30ReadWord();
// 输出结果
printf("CO2: %d ppm, TVOC: %d ppb\r\n", co2, tvoc);
// 延时一段时间再次读取
delay_ms(5000);
}
}
```
需要注意的是,这只是一个简单的示例程序,实际应用中需要根据具体情况进行修改。
阅读全文
相关推荐
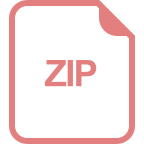

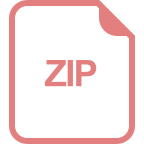
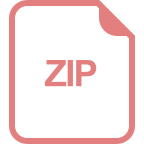
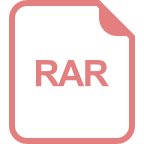
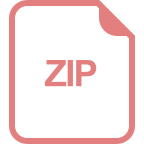
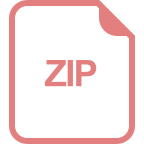
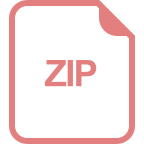
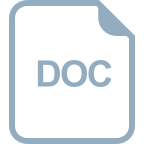
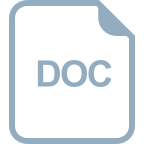
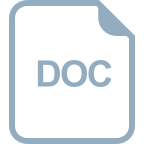
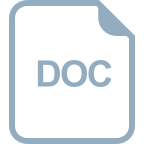
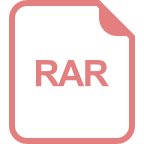
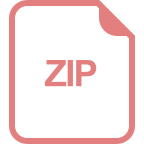
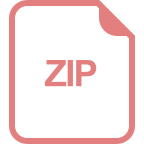