首先,需要定义一个结构体来表示顾客的信息,包括顾客的姓名、身份证号、办理业务类型等。 接着,定义一个队列结构体,用于存储排队的顾客信息。队列结构体需要包括队列长度和队列头、队列尾指针。 定义一个窗口结构体,用于存储窗口的信息,包括窗口号、提供的业务类型、当前服务的顾客等。 在主函数中,首先需要初始化队列和窗口,为每个窗口分配一个业务类型。然后,进入一个循环,等待用户输入命令。 如果用户输入的是到达营业厅的命令,需要创建一个顾客信息,然后将该顾客信息加入到队列中。这里需要注意,需要根据该顾客所办理业务类型,将其加入到队列长度最短的窗口排队。 如果用户输入的是业务办结的命令,需要找到该窗口队列中最先入队的顾客,将其出队,并将该窗口的当前服务顾客更新为下一个入队的顾客。 如果用户输入的是查看排队情况的命令,需要输出各窗口提供的业务类型和当前排队等待人数。 最后,当用户输入退出命令时,程序结束。根据以上思路,用c语言写一个排队系统
时间: 2024-03-05 18:47:32 浏览: 110
好的,我可以给你提供一个排队系统的C语言实现,代码如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_QUEUE_SIZE 10 // 队列最大长度
#define MAX_WINDOW_NUM 5 // 窗口最大数量
#define MAX_NAME_LEN 20 // 姓名最大长度
#define MAX_ID_LEN 20 // 身份证号最大长度
// 顾客信息结构体
typedef struct {
char name[MAX_NAME_LEN];
char id[MAX_ID_LEN];
int service_type;
} Customer;
// 队列结构体
typedef struct {
int length;
int front;
int rear;
Customer queue[MAX_QUEUE_SIZE];
} Queue;
// 窗口结构体
typedef struct {
int window_id;
int service_type;
Customer current_customer;
Queue queue;
} Window;
// 初始化队列
void init_queue(Queue* queue) {
queue->length = 0;
queue->front = 0;
queue->rear = -1;
}
// 判断队列是否为空
int is_empty(Queue* queue) {
return queue->length == 0;
}
// 判断队列是否已满
int is_full(Queue* queue) {
return queue->length == MAX_QUEUE_SIZE;
}
// 入队
void enqueue(Queue* queue, Customer customer) {
if (is_full(queue)) {
printf("The queue is full!\n");
return;
}
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
queue->queue[queue->rear] = customer;
queue->length++;
}
// 出队
Customer dequeue(Queue* queue) {
if (is_empty(queue)) {
printf("The queue is empty!\n");
exit(1);
}
Customer customer = queue->queue[queue->front];
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
queue->length--;
return customer;
}
// 初始化窗口
void init_window(Window* window, int window_id, int service_type) {
window->window_id = window_id;
window->service_type = service_type;
init_queue(&(window->queue));
}
// 分配顾客到队列中
void assign_customer_to_queue(Window* windows, int window_num, Customer customer) {
int shortest_queue_id = 0;
int shortest_length = MAX_QUEUE_SIZE + 1;
for (int i = 0; i < window_num; i++) {
if (windows[i].service_type == customer.service_type && windows[i].queue.length < shortest_length) {
shortest_queue_id = i;
shortest_length = windows[i].queue.length;
}
}
enqueue(&(windows[shortest_queue_id].queue), customer);
}
// 更新窗口服务的顾客信息
void update_window_customer(Window* window) {
if (!is_empty(&(window->queue))) {
window->current_customer = dequeue(&(window->queue));
}
}
// 查看排队情况
void check_queue(Window* windows, int window_num) {
for (int i = 0; i < window_num; i++) {
printf("Window %d - Service Type: %d, Waiting Number: %d\n",
windows[i].window_id, windows[i].service_type, windows[i].queue.length);
}
}
int main() {
Window windows[MAX_WINDOW_NUM];
int window_num = 3;
// 初始化窗口
init_window(&(windows[0]), 1, 1);
init_window(&(windows[1]), 2, 2);
init_window(&(windows[2]), 3, 3);
// 进入循环等待用户输入命令
int running = 1;
while (running) {
printf("\nPlease Input Command:\n");
printf("1. Arrive at the Hall\n");
printf("2. Finish Service\n");
printf("3. Check Queue\n");
printf("4. Quit\n");
int command;
scanf("%d", &command);
switch (command) {
case 1: {
// 到达营业厅
Customer customer;
printf("Please Input Customer Name: ");
scanf("%s", customer.name);
printf("Please Input Customer ID: ");
scanf("%s", customer.id);
printf("Please Input Service Type (1-3): ");
scanf("%d", &(customer.service_type));
assign_customer_to_queue(windows, window_num, customer);
printf("Customer %s Assigned to Queue Successfully!\n", customer.name);
break;
}
case 2: {
// 业务办结
int window_id;
printf("Please Input Window ID: ");
scanf("%d", &window_id);
update_window_customer(&(windows[window_id - 1]));
printf("Window %d Finished Service Successfully!\n", window_id);
break;
}
case 3: {
// 查看排队情况
check_queue(windows, window_num);
break;
}
case 4: {
// 退出程序
running = 0;
break;
}
default: {
printf("Invalid Command!\n");
break;
}
}
}
return 0;
}
```
这个排队系统实现了你所要求的基本功能,包括顾客到达营业厅后分配到队列中,业务办结后更新窗口服务的顾客信息,以及查看排队情况等。你可以根据需要进行修改或者添加其他功能。希望对你有所帮助!
阅读全文
相关推荐
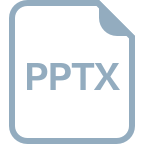
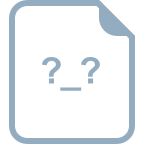
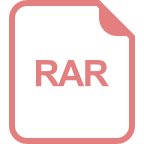















