有个paymentTime,为String类型,为yyyy-MM-dd HH:mm:ss格式,记录了时间信息,在List<Orders> ordersList=ordersService.list()得到总list之后,怎么按照今年的时间划分成12个月的order_price,并用CollUtil.newArrayList将其输出代码简单一点,可以使用hutool工具
时间: 2024-03-26 18:36:21 浏览: 33
使用Hutool工具可以进一步简化代码。可以按照以下方式实现:
```
List<Orders> ordersList = ordersService.list();
double[] monthOrderPrice = new double[12];
for (Orders order : ordersList) {
String paymentTime = order.getPaymentTime();
int month = DateUtil.month(DateUtil.parse(paymentTime)) + 1;
double orderPrice = order.getOrderPrice();
monthOrderPrice[month-1] += orderPrice;
}
List<Double> monthOrderPriceList = ArrayUtil.toList(monthOrderPrice);
System.out.println(monthOrderPriceList);
```
这里使用Hutool的DateUtil工具类,通过parse方法解析paymentTime字符串为Date类型,然后使用month方法获取月份,并将对应月份的订单总价累加。将月份订单总价数组转换为List类型,输出即可。
相关问题
有个paymentTime,为String类型,为yyyy-MM-dd HH:mm:ss格式,记录了时间信息,在List<Orders> ordersList=ordersService.list()得到总list之后,怎么按照今年的时间划分成12个月的order_price,并用CollUtil.newArrayList输出出来
您可以按照以下步骤进行操作:
1. 首先,将List<Orders> ordersList中的订单按照paymentTime字段升序排序,以确保数据是按照时间顺序排列的。
2. 然后,创建一个长度为12的数组monthOrderPrice,用于存储每个月的订单总价。
3. 接下来,遍历ordersList中的每个订单,将订单的总价加入到对应月份的monthOrderPrice数组中。
具体实现方式如下:
```
double[] monthOrderPrice = new double[12];
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
for (Orders order : ordersList) {
Date paymentTime = sdf.parse(order.getPaymentTime());
Calendar calendar = Calendar.getInstance();
calendar.setTime(paymentTime);
int month = calendar.get(Calendar.MONTH);
monthOrderPrice[month] += order.getOrderPrice();
}
```
4. 最后,使用CollUtil.newArrayList方法将monthOrderPrice数组转换为List<Double>类型,并输出即可。
```
List<Double> monthOrderPriceList = CollUtil.newArrayList(monthOrderPrice);
System.out.println(monthOrderPriceList);
```
完整代码如下:
```
List<Orders> ordersList = ordersService.list();
Collections.sort(ordersList, (o1, o2) -> o1.getPaymentTime().compareTo(o2.getPaymentTime()));
double[] monthOrderPrice = new double[12];
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
for (Orders order : ordersList) {
Date paymentTime = sdf.parse(order.getPaymentTime());
Calendar calendar = Calendar.getInstance();
calendar.setTime(paymentTime);
int month = calendar.get(Calendar.MONTH);
monthOrderPrice[month] += order.getOrderPrice();
}
List<Double> monthOrderPriceList = CollUtil.newArrayList(monthOrderPrice);
System.out.println(monthOrderPriceList);
```
vue+springboot的echarts的折线图已经固定好x轴为12个月,y轴为数据库的orders表的按照类型为String,格式为yyyy-MM-dd HH:mm:ss的paymentTime划分的order_price成的每个月的每个订单金额总和,如何实现前端与后端
要实现前端Vue与后端SpringBoot的交互,你需要按如下步骤进行操作:
1. 在SpringBoot中编写API接口,用于查询数据库中订单金额数据并返回给前端。可以使用MyBatis等框架进行数据库操作。
```java
@RestController
@RequestMapping("/api")
public class OrderController {
@Autowired
private OrderMapper orderMapper;
@GetMapping("/orders")
public List<Order> getOrders() {
return orderMapper.selectOrders();
}
}
```
其中,OrderMapper是一个MyBatis的Mapper接口,用于查询数据库中的订单金额数据。
2. 在Vue组件中使用axios等工具调用后端API接口。
```javascript
mounted () {
axios.get('/api/orders').then(res => {
// 处理后端返回的数据
})
}
```
其中,axios.get方法用于向后端API接口发送HTTP GET请求,并在请求成功后执行回调函数。
3. 在Vue组件中处理后端返回的数据,并使用Echarts插件绘制折线图。
```javascript
mounted () {
axios.get('/api/orders').then(res => {
const data = res.data
const xAxisData = [] // x轴数据
const yAxisData = [] // y轴数据
data.forEach(item => {
xAxisData.push(item.month)
yAxisData.push(item.total)
})
const chart = echarts.init(document.getElementById('chart-container'))
const option = {
xAxis: {
type: 'category',
data: xAxisData
},
yAxis: {
type: 'value'
},
series: [{
data: yAxisData,
type: 'line'
}]
}
chart.setOption(option)
})
}
```
其中,res.data是后端API接口返回的数据,可以根据实际情况进行处理。在上面的代码中,我们将数据分别保存到xAxisData和yAxisData数组中,并使用Echarts插件绘制折线图。
阅读全文
相关推荐
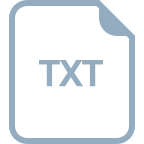
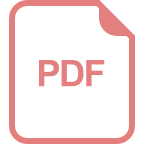
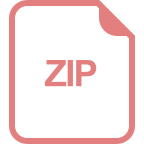
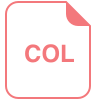
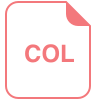
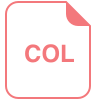
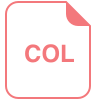
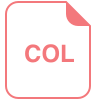
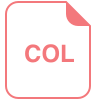
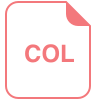
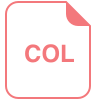
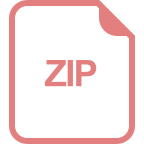