如果一个日期的日期以 1 结尾(1日、11日、21日、31日)且为星期一,则称这个日期为一好日期。 用C语言写一程序计算从 1901 年 1 月 1 日至 2024 年 12 月 31 日总共有多少个一好日期。 提示:1901 年 1 月 1 日是星期二。
时间: 2024-11-19 19:13:08 浏览: 16
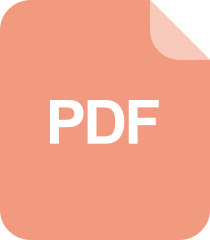
Windows Phone 7 开发31日谈
要编写一个C语言程序来计算从1901年1月1日至2024年12月31日之间的一好日期总数,可以采用以下步骤:
1. 首先,我们需要一个函数来判断给定日期是否是一好日期。这需要对日期进行处理,并检查该日期的星期几。
2. 然后,我们可以遍历1901年至2024年的所有日期,对于每个月,我们找出哪些日期是1日、11日、21日或31日,并检查它们是否是星期一。
3. 计数器用于存储满足条件的一好日期。
下面是一个简单的C程序实现:
```c
#include <stdio.h>
#include <time.h>
int isGoodDate(int day, int month, int year) {
struct tm date;
date.tm_year = year - 1900; // 转换为自1900年以来的年份
date.tm_mon = month - 1; // 减去1,因为月份是从0开始计数的
date.tm_mday = day;
time_t t = mktime(&date); // 将tm结构体转换为时间戳
if (t == -1 || !weekday(t)) { // 检查时间戳是否有效且是星期一
return 0;
}
return (day % 10 == 1 && day != 31); // 只有1日、11日、21日才考虑
}
int main() {
int count = 0;
for (year = 1901; year <= 2024; year++) {
for (month = 1; month <= 12; month++) {
for (day = 1; day <= get_days_in_month(year, month); day++) {
if (isGoodDate(day, month, year)) {
count++;
}
}
}
}
printf("从1901年1月1日至2024年12月31日,总共有%d个一好日期。\n", count);
return 0;
}
// 辅助函数获取某个月份的天数
int get_days_in_month(int year, int month) {
static const int days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (is_leap_year(year)) {
return days[month];
} else {
return days[month - 1]; // February has 28 days in non-leap years
}
}
// 判断是否为闰年
bool is_leap_year(int year) {
return (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0));
}
```
请注意,这个程序没有优化,计算效率较低,因为它会多次检查同一天。如果需要更高效的解决方案,可以考虑在计算每个年份或月份的初始和结束日期时,只检查那些“1”结尾的日子并确定它们是否为星期一。但是上面的版本易于理解。运行此程序后,将得到从1901年到2024年的一好日期总数。
阅读全文
相关推荐
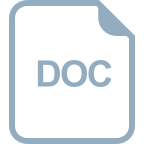
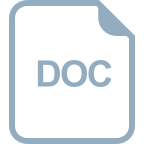









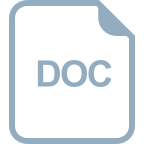
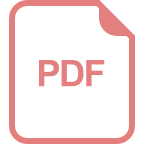
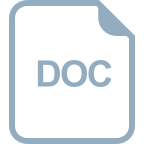
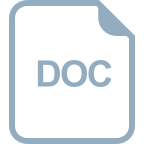
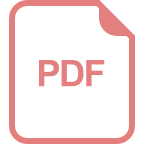
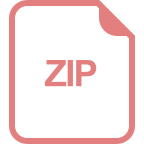
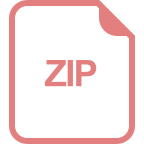
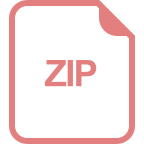