java注解能自定义元注解吗,给一个例子
时间: 2024-05-06 08:20:57 浏览: 9
可以自定义元注解,例如:
```
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface CustomAnnotation {
String value();
}
@Target(ElementType.ANNOTATION_TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface MetaAnnotation {
String value();
}
```
在这个例子中,我们定义了一个自定义注解 `CustomAnnotation`,并定义了一个元注解 `MetaAnnotation`,用于标注在其他注解上。
相关问题
java中自定义注解如何实现注入,给一个例子
好的,关于java中自定义注解的注入实现,一般是通过反射机制来实现的。具体的实现需要遵循一定的规范,首先要定义一个注解,然后在需要使用的地方进行注解的声明,最后在相应的代码中使用反射机制来获取注解信息并实现注入。
以下是一个简单的示例代码:
```
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface TestAnnotation {
String value() default "";
}
public class TestClass {
@TestAnnotation(value = "testValue")
private String testField;
public TestClass() {
// 使用反射获取注解信息
for(Field field : this.getClass().getDeclaredFields()) {
if(field.isAnnotationPresent(TestAnnotation.class)) {
TestAnnotation annotation = field.getAnnotation(TestAnnotation.class);
field.setAccessible(true);
try {
field.set(this, annotation.value());
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
TestClass test = new TestClass();
System.out.println(test.testField); // 输出 "testValue"
}
}
```
这个例子中定义了一个名为 TestAnnotation 的注解,用来注解 TestClass 中的字段。在 TestClass 中,使用反射遍历所有的字段,如果存在 TestAnnotation 的注解,则将注解中的值赋值给该字段。最后在 main 方法中,创建 TestClass 的实例,输出 testField 字段的值。
写一个 java 自定义注解
Java自定义注解又称为元注解,是Java语言的一个特性,可以在Java程序中标记和描述程序元素,包括类、方法、变量、参数等。通过使用自定义注解,可以方便地实现一些自动化处理,例如代码生成、配置文件生成等。
下面是一个简单的Java自定义注解的例子,用于标记方法是否需要进行日志记录。
```
import java.lang.annotation.*;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Loggable {
String value() default "";
}
```
注解的定义使用了`@interface`关键字,注解的名称为`Loggable`。其中,`@Target`注解用于描述注解的作用目标,本例中只能用于方法上,`@Retention`注解用于描述注解的保留期限,本例中指定为运行时保留。
注解中定义了一个`value`属性,用于指定日志信息的描述。如果不指定,则默认为空字符串。
下面是一个使用这个注解的例子。
```
public class MyClass {
@Loggable("MethodA is called")
public void methodA() {
// 方法体
}
@Loggable
public void methodB() {
// 方法体
}
}
```
在上面的例子中,`methodA`方法使用了自定义注解,并指定了日志信息的描述,`methodB`方法使用了自定义注解,但没有指定日志信息的描述。在实际应用中,可以通过反射机制来获取注解信息,从而实现一些自动化处理。
相关推荐
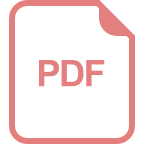












