Java怎么针对判空写一个自定义注解, 给我举个例子
时间: 2024-04-15 18:27:21 浏览: 94
要针对判空写一个自定义注解,你可以使用 Java 的注解和反射机制来实现。下面是一个示例代码,演示如何创建一个自定义的 `@NotNull` 注解来进行非空校验:
首先,创建一个 `NotNull` 注解:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface NotNull {
}
```
在上述代码中,我们使用 `@Retention(RetentionPolicy.RUNTIME)` 注解指定了注解的保留策略为运行时。而 `@Target(ElementType.FIELD)` 注解表示该注解可以用于字段上。
接下来,创建一个测试类来使用 `@NotNull` 注解:
```java
public class Person {
@NotNull
private String name;
public Person(String name) {
this.name = name;
}
public static void main(String[] args) {
Person person1 = new Person("John");
validateNotNullFields(person1);
Person person2 = new Person(null);
validateNotNullFields(person2);
}
private static void validateNotNullFields(Object object) {
for (Field field : object.getClass().getDeclaredFields()) {
if (field.isAnnotationPresent(NotNull.class)) {
field.setAccessible(true);
try {
Object value = field.get(object);
if (value == null) {
throw new IllegalArgumentException("Field '" + field.getName() + "' cannot be null");
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
}
}
```
在上述代码中,我们创建了一个 `Person` 类,并在 `name` 字段上使用了 `@NotNull` 注解。然后,我们在 `main()` 方法中创建了两个 `Person` 对象,一个给了有效的值,另一个给了 `null`。
接着,我们定义了一个 `validateNotNullFields()` 方法,它使用反射来检查对象中带有 `@NotNull` 注解的字段是否为 `null`。如果字段为 `null`,则抛出一个异常。
在上述示例中,`person1` 没有抛出异常,因为 `name` 字段有一个有效的值。而 `person2` 会抛出异常,因为 `name` 字段的值为 `null`。
这只是一个简单的示例,你可以根据自己的需求进行扩展和定制。希望对你有所帮助!
阅读全文
相关推荐
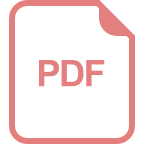
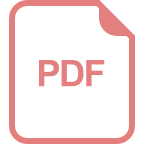
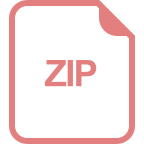















