QString.arg(content)
时间: 2024-04-20 21:24:58 浏览: 87
QString.arg(content)是Qt框架中的一个函数调用,用于将content的值插入到QString对象中的占位符处。具体来说,该函数会在QString对象中查找占位符(例如 %1、%2 等),并将content的值替换到指定的占位符位置上。这样可以方便地构建字符串,将变量或者其他文本内容动态地插入到字符串中。
相关问题
逐步解释下面代码,以及为什么这样写:#include "chatdialog.h" #include "ui_chatdialog.h" #include<QTime> #include<QDebug> ChatDialog::ChatDialog(QWidget *parent) : QDialog(parent), ui(new Ui::ChatDialog) { ui->setupUi(this); } ChatDialog::~ChatDialog() { delete ui; } //设置聊天窗口信息 void ChatDialog::setChatDialogInfo(QString ip) { //保存成员变量 m_ip = ip; //设置窗口title setWindowTitle(QString("与【%1】的聊天窗口").arg(m_ip)); } //设置聊天内容显示到浏览窗口 void ChatDialog::setChatMsg(QString content) { ui->tb_chat->append(QString("【%1】 %2").arg(m_ip).arg(QTime::currentTime().toString("hh:mm:ss"))); ui->tb_chat->append(content); } void ChatDialog::on_pb_send_clicked() { //1.获取到用户输入的纯文本数据并校验,不能是空字符串或者全为空格 QString content = ui->te_chat->toPlainText(); if (content.isEmpty() || content.remove(" ").isEmpty()){ return; } //2.获取带格式的文本,删除框里的内容 content = ui->te_chat->toHtml(); qDebug()<< "content:" <<content; ui->te_chat->clear(); //3.把内容显示到浏览窗口,格式【我】 时间 内容 ui->tb_chat->append(QString("【我】 %1").arg(QTime::currentTime().toString("hh:mm:ss"))); ui->tb_chat->append(content); //4.把ip和内容发给kernel,因为窗口知道,但kernel不知道发给谁 Q_EMIT SIG_sendMsg(content,m_ip); }
这段代码是一个聊天窗口的实现。主要是通过 Qt 框架实现窗口的 UI 设计和交互逻辑。
- `#include "chatdialog.h"`:引入聊天窗口类的头文件。
- `#include "ui_chatdialog.h"`:引入聊天窗口 UI 的头文件。
- `#include<QTime>`:引入 Qt 框架中的时间类。
- `#include<QDebug>`:引入 Qt 框架中的调试输出类。
```cpp
ChatDialog::ChatDialog(QWidget *parent) : QDialog(parent), ui(new Ui::ChatDialog) {
ui->setupUi(this);
}
```
这是聊天窗口的构造函数,其中:
- `QDialog(parent)` 表示调用父类 `QDialog` 的构造函数。
- `ui(new Ui::ChatDialog)` 表示创建一个 `Ui::ChatDialog` 的对象,并将其存储在 `ui` 成员变量中。
- `ui->setupUi(this)` 表示将 UI 设计文件中的控件和信号槽绑定到窗口对象上。
```cpp
ChatDialog::~ChatDialog() {
delete ui;
}
```
这是聊天窗口的析构函数,其中 `delete ui` 表示删除 UI 对象。
```cpp
void ChatDialog::setChatDialogInfo(QString ip) {
m_ip = ip;
setWindowTitle(QString("与【%1】的聊天窗口").arg(m_ip));
}
```
这是设置聊天窗口信息的函数,其中:
- `m_ip = ip` 表示将参数 `ip` 赋值给成员变量 `m_ip`。
- `setWindowTitle(QString("与【%1】的聊天窗口").arg(m_ip))` 表示设置窗口的标题。
```cpp
void ChatDialog::setChatMsg(QString content) {
ui->tb_chat->append(QString("【%1】 %2").arg(m_ip).arg(QTime::currentTime().toString("hh:mm:ss")));
ui->tb_chat->append(content);
}
```
这是将聊天内容显示到浏览窗口的函数,其中:
- `ui->tb_chat->append(QString("【%1】 %2").arg(m_ip).arg(QTime::currentTime().toString("hh:mm:ss")))` 表示在浏览窗口中添加一行显示当前时间和 IP 地址。
- `ui->tb_chat->append(content)` 表示在浏览窗口中添加一行显示聊天内容。
```cpp
void ChatDialog::on_pb_send_clicked() {
QString content = ui->te_chat->toPlainText();
if (content.isEmpty() || content.remove(" ").isEmpty()){
return;
}
content = ui->te_chat->toHtml();
qDebug()<< "content:" <<content;
ui->te_chat->clear();
ui->tb_chat->append(QString("【我】 %1").arg(QTime::currentTime().toString("hh:mm:ss")));
ui->tb_chat->append(content);
Q_EMIT SIG_sendMsg(content,m_ip);
}
```
这是发送聊天内容的函数,其中:
- `ui->te_chat->toPlainText()` 表示获取文本框中的纯文本。
- `content.isEmpty() || content.remove(" ").isEmpty()` 表示判断是否为空字符串或者全为空格,如果是,则不发送。
- `ui->te_chat->toHtml()` 表示获取文本框中带格式的文本。
- `ui->te_chat->clear()` 表示清空文本框中的内容。
- `ui->tb_chat->append(QString("【我】 %1").arg(QTime::currentTime().toString("hh:mm:ss")))` 表示在浏览窗口中添加一行显示当前时间和发送人为“我”。
- `ui->tb_chat->append(content)` 表示在浏览窗口中添加一行显示聊天内容。
- `Q_EMIT SIG_sendMsg(content,m_ip)` 表示发出信号,通知其他模块发送了消息。
qstring如何获取双引号间的内容
QString是一个Qt库中的字符串类,在C++中,如果你想从包含双引号(")的QString中提取内容,特别是如果该内容本身也包含了引号作为文本的一部分,你需要特别注意解析。如果你直接尝试使用`.`或`[]`访问,它可能会遇到困扰。
通常的做法是使用`QString::arg()`函数,或者使用`substr()`和正则表达式。例如:
```cpp
// 如果你知道确切的位置
QString str = "这是一个 \"包含双引号\" 的字符串";
QString content = str.mid(str.indexOf("\"") + 1, str.indexOf("\"", str.indexOf("\"") + 1) - str.indexOf("\"") - 1);
// 或者使用find()和多次substring()
int startQuoteIndex = str.indexOf("\"");
if (startQuoteIndex != -1) {
int endQuoteIndex = str.indexOf("\"", startQuoteIndex + 1);
if (endQuoteIndex != -1) {
content = str.mid(startQuoteIndex + 1, endQuoteIndex - startQuoteIndex - 1);
}
}
// 使用正则表达式更安全,避免嵌套引号的问题
QRegExp regex("\"([^\"]*)\"");
QMatch match = regex.match(str);
if (match.hasMatch()) {
content = match.captured(1);
}
```
这里的关键点是找到开始和结束的引号位置,并确保它们之间没有其他成对的引号导致错误。
阅读全文
相关推荐
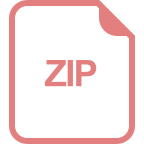
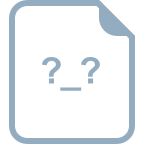
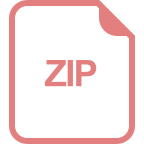
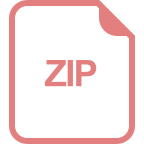
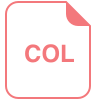

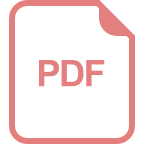
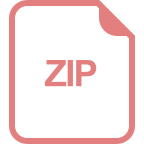
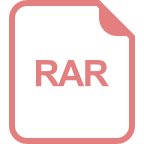
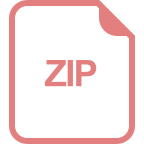
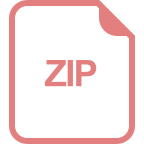
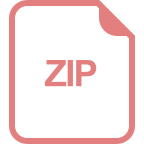
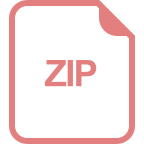
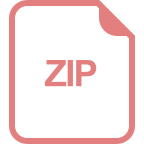
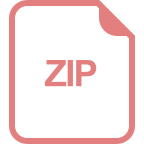
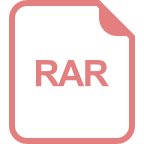