输入一个含字母、数字、空格、和其他字符的字符串,统计这个字符串中的字母、数字、空格以及其他字符的个数
时间: 2023-11-19 18:00:45 浏览: 43
好的,我可以帮你解决这个问题。这里有一个 Python 的函数可以完成这个任务:
```python
def count_chars(s):
"""
统计字符串中字母、数字、空格和其他字符的个数
"""
letter_count = 0
digit_count = 0
space_count = 0
other_count = 0
for c in s:
if c.isalpha():
letter_count += 1
elif c.isdigit():
digit_count += 1
elif c.isspace():
space_count += 1
else:
other_count += 1
return letter_count, digit_count, space_count, other_count
```
这个函数使用了一个 for 循环,遍历了字符串中的每个字符。然后,它使用了字符串的 isalpha()、isdigit() 和 isspace() 方法来判断每个字符属于哪一类。最后,函数返回了每一类字符的个数,作为一个元组。你可以调用这个函数来实现你的需求。
相关问题
请用C语言编程输入一个含字母、数字、空格、和其他字符的字符串,统计这个字符串中的字母、数字、空格以及其他字符的个数
以下是用C语言编写的代码,实现了输入一个字符串,统计其中字母、数字、空格和其他字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, letters=0, digits=0, spaces=0, others=0;
printf("请输入一个字符串:");
gets(str);
for (i = 0; str[i]!='\0'; i++) {
if (isalpha(str[i]))
letters++;
else if (isdigit(str[i]))
digits++;
else if (isspace(str[i]))
spaces++;
else
others++;
}
printf("字母个数:%d\n", letters);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
代码解释如下:
1. 定义一个字符数组 `str`,用于存储输入的字符串,最大长度为100。
2. 定义四个整型变量 `letters`、`digits`、`spaces` 和 `others`,分别用于统计字母、数字、空格和其他字符的个数,初始值都为0。
3. 使用 `gets()` 函数从标准输入(即键盘)读入一个字符串,存储到 `str` 数组中。
4. 使用 for 循环遍历字符串 `str` 中的每一个字符,如果是字母,则 `letters` 计数器加1,如果是数字,则 `digits` 计数器加1,如果是空格,则 `spaces` 计数器加1,否则认为是其他字符,`others` 计数器加1。
5. 最后输出统计结果,即各种字符的个数。
注意:这里使用了 `gets()` 函数,可以读取包含空格的字符串,但是该函数不安全,因为它不会检查字符串长度,可能会导致缓冲区溢出。实际开发中应该使用更安全的函数,例如 `fgets()`。
编写一个函数由实参传来一个字符串统计此字符串中字母数字空格和其他字符的个数在主函数中输入字符串以及输出上述的结果
以下是 Python 代码实现:
```python
def count_chars(string):
letters = 0
digits = 0
spaces = 0
others = 0
for char in string:
if char.isalpha():
letters += 1
elif char.isdigit():
digits += 1
elif char.isspace():
spaces += 1
else:
others += 1
print("字母个数:", letters)
print("数字个数:", digits)
print("空格个数:", spaces)
print("其他字符个数:", others)
# 主函数
if __name__ == '__main__':
string = input("请输入一个字符串: ")
count_chars(string)
```
示例输出:
```
请输入一个字符串: Hello World! 123
字母个数: 10
数字个数: 3
空格个数: 2
其他字符个数: 1
```
阅读全文
相关推荐
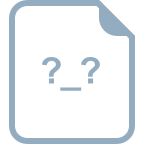












