编程:编写一程序(应该有多个函数),允许从键盘输入任意多个英语单词(单词可以重复),中间用空格分开,输入0表示输入结束。该程序可以统计同一个英语单词被输入几次,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。(提示,尝试用结构体组织数据,把单词和该单出现的次数用一个结构体来描述。
时间: 2023-05-29 21:04:58 浏览: 115
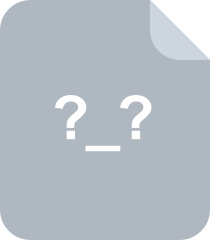
编写一个程序,用于统计文件中单词的总数,不同单词的数目。(假设输入文件中只包含字母和空格)

#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORD_LENGTH 50
#define MAX_NUM_WORDS 1000
typedef struct {
char word[MAX_WORD_LENGTH];
int count;
} WordCount;
void strip(char *word) {
int i = 0, j = strlen(word) - 1;
while (i <= j && isspace(word[i])) {
i++;
}
while (j >= i && isspace(word[j])) {
j--;
}
memmove(word, word + i, j - i + 1);
word[j - i + 1] = '\0';
}
int compare(const void *a, const void *b) {
const WordCount *wordCountA = (const WordCount *)a;
const WordCount *wordCountB = (const WordCount *)b;
return strcmp(wordCountA->word, wordCountB->word);
}
int main() {
char input[MAX_WORD_LENGTH];
WordCount words[MAX_NUM_WORDS];
int numWords = 0;
printf("Please enter words, separated by spaces:\n");
while (1) {
scanf("%s", input);
if (strcmp(input, "0") == 0) {
break;
}
strip(input);
if (strlen(input) == 0) {
continue;
}
int found = 0;
for (int i = 0; i < numWords; i++) {
if (strcmp(words[i].word, input) == 0) {
words[i].count++;
found = 1;
break;
}
}
if (!found) {
WordCount newWordCount = {
.count = 1
};
strcpy(newWordCount.word, input);
words[numWords] = newWordCount;
numWords++;
}
}
qsort(words, numWords, sizeof(WordCount), compare);
printf("Words (sorted alphabetically) and their counts:\n");
for (int i = 0; i < numWords; i++) {
printf("%s: %d\n", words[i].word, words[i].count);
}
return 0;
}
阅读全文
相关推荐
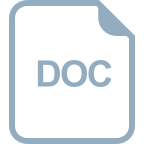
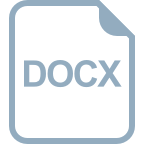
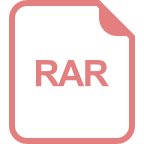
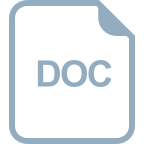
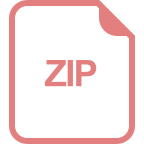
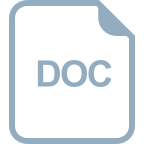
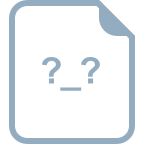
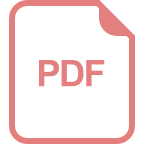
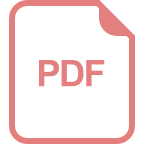
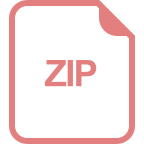
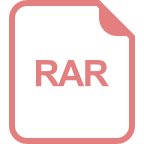
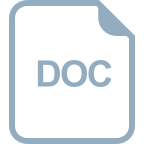
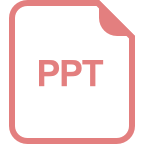
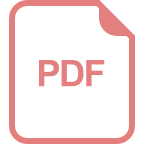
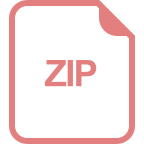
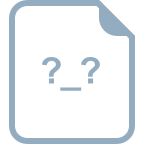
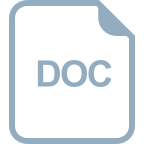