C语言或者c++中如何计算一个对象所占的空间大小
时间: 2024-01-15 22:05:14 浏览: 75
在 C 或 C++ 中,计算一个对象所占用的空间大小相对比较简单。
对于 C 语言,我们可以使用 `sizeof` 运算符来计算一个对象的大小,例如:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
int a;
printf("Size of int: %ld bytes\n", sizeof(a));
return 0;
}
```
上述代码将输出 `Size of int: 4 bytes`,表示 `int` 类型所占用的空间大小为 4 个字节。
对于 C++ 语言,我们同样可以使用 `sizeof` 运算符来计算一个对象的大小,例如:
```c++
#include <iostream>
using namespace std;
int main() {
int a;
cout << "Size of int: " << sizeof(a) << " bytes" << endl;
return 0;
}
```
上述代码将输出 `Size of int: 4 bytes`,表示 `int` 类型所占用的空间大小为 4 个字节。
需要注意的是,对于结构体和类等复杂类型的对象,其大小取决于其成员变量的类型和数量,需要逐个计算并累加,可以使用 `sizeof` 运算符和 `offsetof` 宏来计算。例如:
```c++
#include <iostream>
#include <cstddef>
using namespace std;
struct Person {
string name;
int age;
};
int main() {
Person p;
cout << "Size of Person: " << sizeof(p) << " bytes" << endl;
cout << "Offset of name: " << offsetof(Person, name) << " bytes" << endl;
cout << "Offset of age: " << offsetof(Person, age) << " bytes" << endl;
return 0;
}
```
上述代码将输出 `Size of Person: 16 bytes`,表示 `Person` 类型所占用的空间大小为 16 个字节,其中 `name` 字符串占用 8 个字节,`age` 整数占用 4 个字节,剩余 4 个字节为内存对齐所需的填充字节。
阅读全文
相关推荐
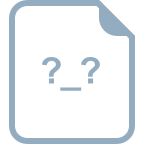
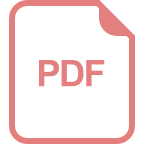
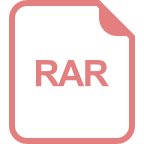
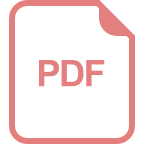
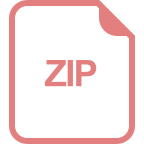
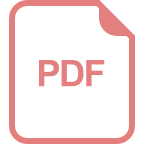
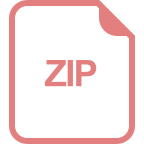
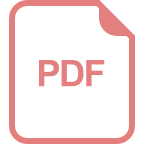
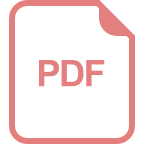
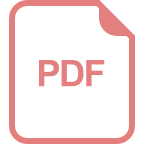
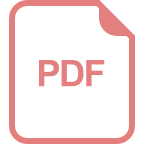
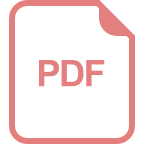
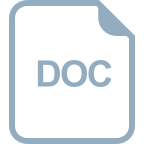
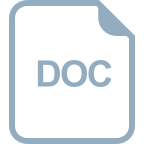
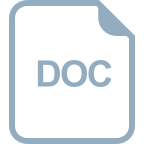
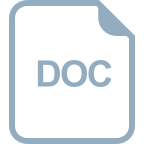
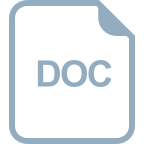
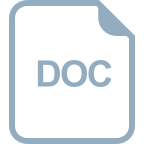