python 爬虫代码
时间: 2023-09-16 18:05:38 浏览: 145
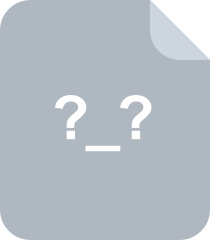
爬虫_python_一款更新抓取one文艺网站的代码的程序
### 回答1:
以下是一个简单的 Python 爬虫代码示例,使用 requests 和 BeautifulSoup 库:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
# 找到页面中所有的链接
links = []
for link in soup.find_all("a"):
links.append(link.get("href"))
# 输出所有链接
for link in links:
print(link)
```
以上代码会爬取给定网页的所有链接,并输出到控制台。如果需要获取更多的信息,可以根据网页的 HTML 结构,使用 BeautifulSoup 进行解析。注意:在进行网络爬取时,请确保遵守网站的 robots.txt 文件,以避免对网站造成不必要的压力或损害。
### 回答2:
爬虫是一种自动化获取互联网数据的技术,而Python是一种简洁、易读的编程语言,因此Python常被用来编写爬虫代码。下面是一个简单的Python爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 定义要爬取的目标网页链接
url = "https://example.com"
# 发送HTTP GET请求,获取目标网页的内容
response = requests.get(url)
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.text, "html.parser")
# 提取目标数据
title = soup.title.text
paragraphs = soup.find_all("p")
# 打印提取结果
print("标题: ", title)
print("段落数: ", len(paragraphs))
for paragraph in paragraphs:
print(paragraph.text)
```
上述代码使用了requests库发送HTTP GET请求,并使用BeautifulSoup库解析网页内容。首先,我们指定要爬取的目标网页链接,并使用requests.get()方法获取网页的内容。然后,我们使用BeautifulSoup处理网页内容,通过提取title标签的文本和找到所有的段落<p>标签,我们得到了目标数据。最后,将结果打印出来。
当然,这只是一个简单的示例,实际的爬虫代码可能会复杂得多,可能会需要处理javascript渲染的页面、处理验证码、使用代理等操作。但这个示例可以作为入门学习爬虫的起点。
### 回答3:
爬虫是一种自动化的程序,在互联网上获取信息。Python是一种非常适合编写爬虫的编程语言。下面是一个用Python编写的简单爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 定义一个函数,用于获取指定URL的页面内容
def get_html(url):
try:
response = requests.get(url)
response.raise_for_status() # 若请求出错,则抛出异常
response.encoding = response.apparent_encoding
return response.text
except Exception as e:
print("获取页面内容失败:", e)
return None
# 定义一个函数,用于解析页面内容,提取所需信息
def parse_html(html):
try:
soup = BeautifulSoup(html, 'html.parser')
# 在这里编写解析代码,提取需要的信息
# 例如,查找所有的 <a> 标签并获取链接
links = soup.find_all('a')
for link in links:
print(link['href'])
except Exception as e:
print("解析页面内容失败:", e)
# 主函数入口
if __name__ == '__main__':
url = 'http://www.example.com'
html = get_html(url)
if html:
parse_html(html)
```
以上代码首先导入了requests库和BeautifulSoup库。使用requests库发送HTTP请求获取网页内容,使用BeautifulSoup库解析网页内容。
该代码中定义了两个函数:`get_html`用于获取指定URL的页面内容,`parse_html`用于解析页面内容,提取所需信息。
在主函数中,先指定要爬取的网页URL,然后使用`get_html`函数获取页面内容,并将内容传递给`parse_html`函数进行解析。
以上代码只是一个简单的示例,实际的爬虫代码会根据具体需求进行更复杂的处理和数据提取。
阅读全文
相关推荐
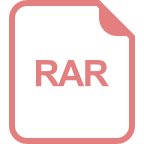
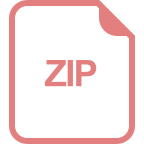
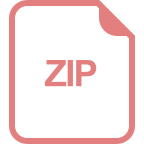
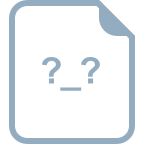
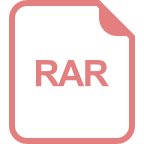
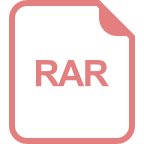
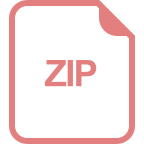
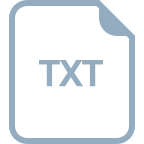