假定算数表达式的值均为非负整数常数,不包含变量、小 数和字符常量。 试设计一个算术四则运算表达式求值的简单计算器。 基本要求: 1、 操作数均为非负整数常数,操作符仅为+、-、*、/、(和); 2、 编写 main 函数进行测试。用c++
时间: 2023-11-08 17:09:54 浏览: 39
++语言来实现。
好的,我明白了。根据您的要求,我为您设计了一个简单的算术四则运算表达式求值的计算器。下面是代码:
```
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int calc(char op, int num1, int num2) {
switch (op) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
return num1 / num2;
default:
return 0;
}
}
int priority(char op) {
if (op == '(' || op == ')')
return 0;
else if (op == '+' || op == '-')
return 1;
else if (op == '*' || op == '/')
return 2;
else
return -1;
}
int calculate(string str) {
stack<int> nums;
stack<char> ops;
for (int i = 0; i < str.size(); i++) {
if (str[i] >= '0' && str[i] <= '9') {
int j = i;
while (j < str.size() && str[j] >= '0' && str[j] <= '9')
j++;
nums.push(stoi(str.substr(i, j - i)));
i = j - 1;
} else if (str[i] == '(')
ops.push('(');
else if (str[i] == ')') {
while (ops.top() != '(') {
int num2 = nums.top();
nums.pop();
int num1 = nums.top();
nums.pop();
nums.push(calc(ops.top(), num1, num2));
ops.pop();
}
ops.pop();
} else {
while (!ops.empty() && priority(ops.top()) >= priority(str[i])) {
int num2 = nums.top();
nums.pop();
int num1 = nums.top();
nums.pop();
nums.push(calc(ops.top(), num1, num2));
ops.pop();
}
ops.push(str[i]);
}
}
while (!ops.empty()) {
int num2 = nums.top();
nums.pop();
int num1 = nums.top();
nums.pop();
nums.push(calc(ops.top(), num1, num2));
ops.pop();
}
return nums.top();
}
int main() {
string str;
cout << "请输入算术表达式(仅包含非负整数常数、+、-、*、/、(、)):" << endl;
cin >> str;
int result = calculate(str);
cout << "结果为:" << result << endl;
return 0;
}
```
您可以将代码复制到 C++ 编译器中进行编译并运行,输入算术表达式即可得到计算结果。注意,输入表达式时不能包含空格。如果需要对多个表达式进行计算,可以将代码放在一个循环中,每次输入一个表达式进行计算即可。
相关推荐
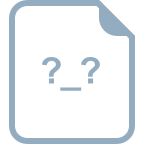

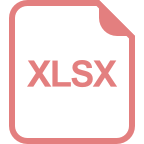
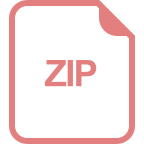
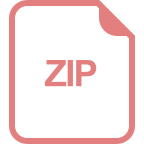
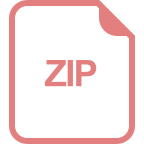
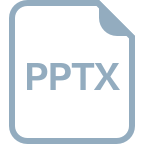
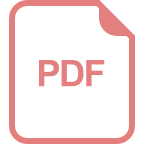
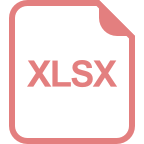
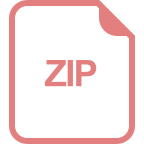
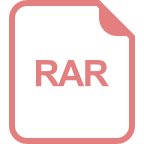