请实现一个MyQueue类,实现出队,入队,求队列长度. 实现入队函数 void push(int x); 实现出队函数 int pop(); 实现求队列长度函数 int size();
时间: 2024-10-24 19:03:45 浏览: 75
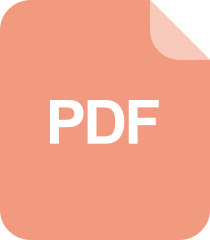
C++利用两个栈实现队列的方法
```cpp
#include <iostream>
using namespace std;
class MyQueue {
private:
int* queue; // 队列数组
int front, rear; // 栈顶和栈底指针
int capacity; // 容量
public:
// 构造函数,初始化队列为空
MyQueue(int size) {
this->capacity = size;
front = rear = -1;
queue = new int[capacity];
}
// 函数实现入队操作
void push(int x) {
if ((rear + 1) % capacity == front) { // 判断队满
cout << "Queue is full.\n";
return;
}
rear = (rear + 1) % capacity; // 先进后出原则,更新后端指针
queue[rear] = x;
cout << "Element " << x << " pushed to the queue.\n";
}
// 函数实现出队操作
int pop() {
if (front == -1) { // 判断队空
cout << "Queue is empty.\n";
return -1;
}
int item = queue[front]; // 取出前端元素
queue[front] = -1; // 简化队列,设置前端为空标记
front = (front + 1) % capacity; // 更新前端指针
cout << "Popped element from the queue: " << item << "\n";
return item;
}
// 函数实现求队列长度
int size() {
if (front > rear) {
return rear - front + 1; // 如果front大于rear,则有效元素个数为两者之差加1
} else {
return capacity - front; // 否则,有效元素个数为总容量减去front
}
}
~MyQueue() { // 析构函数,释放内存
delete[] queue;
}
};
int main() {
MyQueue mq(5); // 创建一个大小为5的队列
mq.push(1);
mq.push(2);
mq.push(3);
cout << "Queue length: " << mq.size() << endl;
mq.pop();
mq.pop();
cout << "Queue length after popping two elements: " << mq.size() << endl;
return 0;
}
```
在这个示例中,`MyQueue` 类实现了基本的入队、出队和获取队列长度功能。注意这里我们使用了循环数组来简化队列的操作,并通过 `front` 和 `rear` 指针记录队列状态。队列满了时提示“Queue is full”,队列空时提示“Queue is empty”。
阅读全文
相关推荐
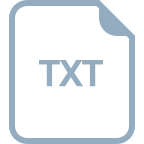
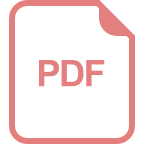


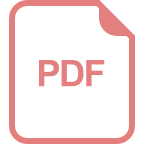
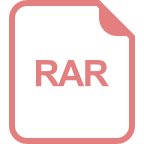
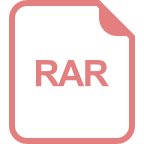
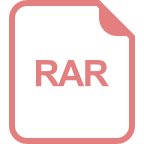
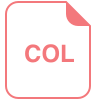
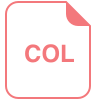
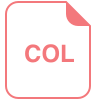
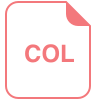





