请实现一个myqueue类,实现出队,入队,求队列长度. 实现入队函数 void push(int x); 实现出队函数 int pop(); 实现求队列长度函数 int size();
时间: 2023-05-31 14:17:57 浏览: 332
### 回答1:
以下是一种可以使用数组实现的队列类的C++代码:
```
class MyQueue {
public:
MyQueue() {
head = tail = 0;
}
void push(int x) {
data[tail++] = x;
}
int pop() {
return data[head++];
}
int size() {
return tail - head;
}
private:
int data[10001];
int head, tail;
};
```
这是一个简单的队列实现,其中数组的大小设置为10001,实际应用中应该根据需要动态调整。
### 回答2:
题目要求我们实现一个myqueue类,实现出队,入队和求队列长度的功能。先来看一下队列的定义:
队列(Queue)是一种先进先出(First In First Out,FIFO)的数据结构,它只能在队尾插入元素,在队首删除元素。在具体实现中,一般是通过链表或者数组来存储元素。
在本题中,我们可以选择用数组来实现队列。因为数组的特点是访问任意一个元素的时间复杂度为O(1),而且在实现时比较简单。
首先我们需要定义一个myqueue类,包含成员变量和成员函数。其中,成员变量用于存储队列中的元素,成员函数用于实现队列的基本操作。
```cpp
class MyQueue {
private:
int q[100]; // 队列的数组
int front; // 队首指针
int rear; // 队尾指针
public:
MyQueue(); // 构造函数
void push(int x); // 入队函数
int pop(); // 出队函数
int size(); // 求队列长度函数
};
```
其中,构造函数用于初始化队列的头指针和尾指针:
```cpp
MyQueue::MyQueue() {
front = rear = 0; // 头指针和尾指针都指向0
}
```
入队函数需要实现对队列尾指针的更新:
```cpp
void MyQueue::push(int x) {
q[rear++] = x; // 将x添加到队尾,同时更新队尾指针rear
}
```
注意:当队列满了时,我们需要判断队列是否已满,如果队列已满则不能再插入新的元素。
出队函数需要实现对队列头指针的更新:
```cpp
int MyQueue::pop() {
int x = q[front++]; // 取出队首元素,同时更新队首指针front
return x;
}
```
注意:当队列为空时,我们需要判断队列是否已空,如果队列已空则不能再取出元素。
求队列长度的函数很简单,只需要返回队尾指针减去队首指针的值即可:
```cpp
int MyQueue::size() {
return rear - front;
}
```
最终的代码如下:
```cpp
class MyQueue {
private:
int q[100]; // 队列的数组
int front; // 队首指针
int rear; // 队尾指针
public:
MyQueue(); // 构造函数
void push(int x); // 入队函数
int pop(); // 出队函数
int size(); // 求队列长度函数
};
// 构造函数
MyQueue::MyQueue() {
front = rear = 0; // 头指针和尾指针都指向0
}
// 入队函数
void MyQueue::push(int x) {
q[rear++] = x; // 将x添加到队尾,同时更新队尾指针rear
}
// 出队函数
int MyQueue::pop() {
int x = q[front++]; // 取出队首元素,同时更新队首指针front
return x;
}
// 求队列长度函数
int MyQueue::size() {
return rear - front;
}
```
这样,我们就完成了本题的要求,可以在程序中创建MyQueue类的对象,通过调用成员函数来操作队列,实现入队、出队、求队列长度等功能。
### 回答3:
队列是一种线性数据结构,具有先进先出(FIFO)的特点。实现一个myqueue类,实现出队,入队,求队列长度。
首先我们需要定义一个队列的结构体,用来存储队列的元素和队首位置,队列长度等信息。
```
struct Queue {
int data[1000];
int front, rear;
};
```
接着,我们需要实现三个函数。
1. 入队函数void push(int x):将元素x插入队列的末尾,同时更新队列长度和队尾位置。
```
void push(int x) {
q.data[q.rear++] = x;
q.length++;
}
```
2. 出队函数int pop():将队首元素弹出,同时更新队列长度和队首位置。
```
int pop() {
q.length--;
return q.data[q.front++];
}
```
3. 求队列长度函数int size():返回队列长度。
```
int size() {
return q.length;
}
```
最终,完整的myqueue类代码如下:
```
#include <iostream>
using namespace std;
struct Queue {
int data[1000];
int front, rear, length;
Queue() {
front = rear = length = 0;
}
};
class MyQueue {
public:
Queue q;
void push(int x) {
q.data[q.rear++] = x;
q.length++;
}
int pop() {
q.length--;
return q.data[q.front++];
}
int size() {
return q.length;
}
};
int main() {
MyQueue q;
q.push(1);
q.push(2);
q.push(3);
cout << q.pop() << endl; // 1
cout << q.pop() << endl; // 2
cout << q.size() << endl; // 1
return 0;
}
```
可以看到,我们成功地实现了出队,入队,求队列长度的功能。
阅读全文
相关推荐
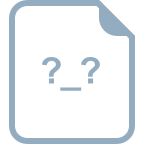



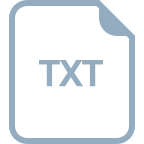
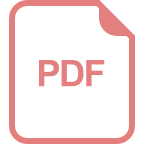
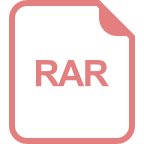
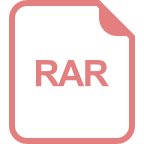
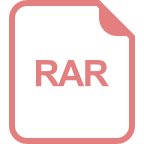
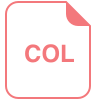
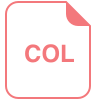
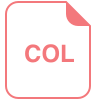
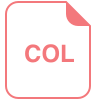



