Cv2.FindContours(image, out var allContours, out _, RetrievalModes.List, ContourApproximationModes.ApproxSimple); OpenCVException: Unrecognized or unsupported array type OpenCvSharp.NativeMethods+<>c.<.cctor>b__1332_0 (OpenCvSharp.ErrorCode status, System.String funcName, System.String errMsg, System.String fileName, System.Int32 line, System.IntPtr userdata) (at <8745c248865442b0b751b51a12326060>:0) (wrapper native-to-managed) OpenCvSharp.NativeMethods+<>c.<.cctor>b__1332_0(OpenCvSharp.ErrorCode,intptr,intptr,intptr,int,intptr) OpenCvSharp.Cv2.FindContours (OpenCvSharp.InputOutputArray image, OpenCvSharp.Point[][]& contours, OpenCvSharp.HierarchyIndex[]& hierarchy, OpenCvSharp.RetrievalModes mode, OpenCvSharp.ContourApproximationModes method, System.Nullable`1[T] offset) (at <8745c248865442b0b751b51a12326060>:0)
时间: 2024-04-13 12:29:10 浏览: 147
根据您提供的代码和错误信息,问题可能出在输入图像的数组类型上。函数 `Cv2.FindContours` 要求输入的图像是 OpenCV 支持的特定数组类型,而您的输入图像可能不符合要求。
要解决这个问题,您可以尝试将输入图像转换为正确的数组类型。例如,您可以使用 `Cv2.CvtColor` 函数将图像转换为灰度图像,然后再应用 `Cv2.FindContours`。下面是一个示例代码:
```csharp
using OpenCvSharp;
// 读取图像
Mat image = Cv2.ImRead("image.jpg", ImreadModes.Color);
// 将图像转换为灰度图像
Mat grayImage = new Mat();
Cv2.CvtColor(image, grayImage, ColorConversionCodes.BGR2GRAY);
// 查找轮廓
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(grayImage, out contours, out hierarchy, RetrievalModes.List, ContourApproximationModes.ApproxSimple);
// 处理轮廓
// ...
```
请注意,这只是一个示例,具体的解决方法可能因您的实际情况而有所不同。如果问题仍然存在,请提供更多关于您的代码和输入图像的信息,以便更好地帮助您解决问题。
相关问题
// 查找图像中的轮廓线 OpenCvSharp.Point[][] allContours = Cv2.FindContoursAsArray(grayImage, RetrievalModes.External, ContourApproximationModes.ApproxSimple); OpenCVException: Unrecognized or unsupported array type
如果您在使用 `Cv2.FindContoursAsArray` 函数时遇到了 `OpenCVException: Unrecognized or unsupported array type` 的错误,这可能是由于输入的图像类型不受支持导致的。
`Cv2.FindContoursAsArray` 函数要求输入的图像是二值图像(例如灰度图像或二进制图像),而不是彩色图像。请确保您的 `grayImage` 是正确的灰度图像或二值图像。
如果 `grayImage` 是彩色图像,您需要先将其转换为灰度图像或二值图像。以下是一个示例代码,演示如何将彩色图像转换为灰度图像并提取轮廓线:
```csharp
using OpenCvSharp;
// 读取彩色图像
Mat colorImage = Cv2.ImRead("image.png", ImreadModes.Color);
// 将图像转换为灰度图像
Mat grayImage = new Mat();
Cv2.CvtColor(colorImage, grayImage, ColorConversionCodes.BGR2GRAY);
// 阈值化处理
Mat binaryImage = new Mat();
Cv2.Threshold(grayImage, binaryImage, 127, 255, ThresholdTypes.Binary);
// 查找轮廓线
Point[][] contours = Cv2.FindContoursAsArray(binaryImage, RetrievalModes.External, ContourApproximationModes.ApproxSimple);
// 处理轮廓线
// ...
```
在示例代码中,我们首先使用 `Cv2.CvtColor` 函数将彩色图像 `colorImage` 转换为灰度图像 `grayImage`。然后,我们使用阈值化处理将灰度图像转换为二值图像 `binaryImage`。最后,我们使用 `Cv2.FindContoursAsArray` 函数查找轮廓线。
请确保在调用 `Cv2.FindContoursAsArray` 函数之前,您的图像已经正确转换为灰度图像或二值图像。
linaro@bm1684:/data/yolact/python$ python3 yolact_bmcv.py --cfgfile configs/yolact_base.cfg--model ../data/models/yolact_base_54_800000_fp32_b1.bmodel --input_path ../data/images usage: yolact_bmcv.py [-h] [--cfgfile CFGFILE] [--model MODEL] [--dev_id DEV_ID] [--thresh THRESH] [--nms NMS] [--keep KEEP] [--is_video IS_VIDEO] [--input_path INPUT_PATH] [--output_dir OUTPUT_DIR] yolact_bmcv.py: error: unrecognized arguments: ../data/models/yolact_base_54_800000_fp32_b1.bmodel
这个命令看起来有几个参数没有正确设置,导致出现了错误。建议您检查一下命令中的参数是否有误,确保每个参数之间有正确的空格或其他分隔符。以下是一些可能需要检查的问题:
1. 您的命令中是否正确设置了 `--cfgfile` 参数和 `--model` 参数?如果这些参数没有正确设置,那么程序将无法找到配置文件和模型文件,从而导致错误。
2. 您的命令中是否正确设置了 `--input_path` 参数?如果这个参数没有正确设置,那么程序将无法找到输入图像,也会导致错误。
3. 您的命令中是否正确设置了 `--output_dir` 参数?如果这个参数没有正确设置,那么程序将无法将处理后的图像保存到正确的输出目录中。
4. 您的命令中是否正确设置了其他参数,如 `--thresh`、 `--nms`、 `--keep`、 `--is_video` 等?如果这些参数没有正确设置,那么程序可能无法以预期的方式运行。
您可以尝试使用 `python3 yolact_bmcv.py -h` 命令来查看该程序的帮助文档,了解各个参数的具体含义和用法,以便更好地进行调试和排错。
阅读全文
相关推荐
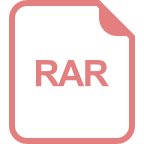
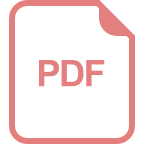
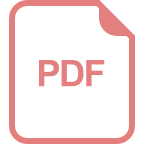














