用C语言实现哈希表存储单词,并且对其进行查找,插入,删除,等操作
时间: 2024-12-21 12:25:27 浏览: 32
在C语言中,实现一个简单的哈希表用于存储单词可以利用数组和链表相结合的方式。这里我们将创建一个哈希函数、桶(数组元素)和链表结构来存储单词。
首先,定义一个结构体来存储单词及其相关信息:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char* word;
int frequency; // 如果需要记录频率,可以用整数
struct Node* next;
} Node;
typedef struct HashTable {
const int size;
Node** buckets;
} HashTable;
```
接下来,我们需要实现哈希函数,通常通过取单词首字母的ASCII码除以哈希表大小并向下取整来确定索引:
```c
int hash(char* key, int size) {
return (int)key[0] % size;
}
```
然后,初始化哈希表:
```c
HashTable* createHashTable(int size) {
HashTable* table = (HashTable*)malloc(sizeof(HashTable));
table->size = size;
table->buckets = (Node**)calloc(size, sizeof(Node*));
for (int i = 0; i < size; i++) {
table->buckets[i] = NULL;
}
return table;
}
void insertIntoHashTable(HashTable* table, char* word) {
int index = hash(word, table->size);
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->word = strdup(word);
newNode->next = table->buckets[index];
table->buckets[index] = newNode;
}
```
查找和删除操作则可以遍历链表:
```c
char* searchHashTable(HashTable* table, char* word) {
int index = hash(word, table->size);
Node* current = table->buckets[index];
while (current != NULL) {
if (strcmp(current->word, word) == 0) {
return current->word;
}
current = current->next;
}
return NULL; // 如果未找到,则返回NULL
}
void deleteFromHashTable(HashTable* table, char* word) {
int index = hash(word, table->size);
Node* current = table->buckets[index], *prev = NULL;
while (current != NULL && strcmp(current->word, word) != 0) {
prev = current;
current = current->next;
}
if (current != NULL) {
if (prev == NULL) {
table->buckets[index] = current->next;
} else {
prev->next = current->next;
}
free(current);
}
}
```
请注意,这个实现是一个简化的示例,实际应用中可能需要考虑负载因子、冲突解决策略(如开放寻址或链地址法)以及内存管理等问题。
阅读全文
相关推荐









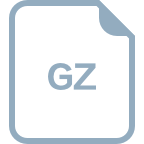








