gpio_operation
时间: 2024-08-16 20:04:41 浏览: 62
GPIO (General Purpose Input/Output)操作通常指的是在嵌入式系统开发中对数字输入输出信号进行控制的过程。在Python中,通过`RPi.GPIO`库可以方便地进行GPIO操作,如读取和设置GPIO状态。以下是一个简单的GPIO操作示例:
```python
from RPi import GPIO
# 设置GPIO模式(BCM编码)
GPIO.setmode(GPIO.BCM)
# 选择一个GPIO针作为输出,比如GPIO 17
pin_number = 17
GPIO.setup(pin_number, GPIO.OUT)
# 设置GPIO为高电平(亮灯)
GPIO.output(pin_number, True)
print(f"Pin {pin_number} set to HIGH")
# 或者设置为低电平(熄灯)
GPIO.output(pin_number, False)
print(f"Pin {pin_number} set to LOW")
# 清理GPIO资源
GPIO.cleanup()
```
在这个例子中,我们设置了GPIO 17为输出模式,然后可以通过`GPIO.output()`函数将其驱动为高电平或低电平。完成操作后,记得调用`GPIO.cleanup()`来释放资源。
相关问题
_WritePin(GPIOI, GPIO_PIN_8, GPIO_PIN_RESET); //HAL_GPIO_TogglePin(GPIOI, GPIO_PIN_8); //HAL_GPIO_TogglePin(GPIOC, GPIO_PIN_15);
The provided code snippets are in the context of the HAL (Hardware Abstraction Layer) for the STM32 microcontroller family, specifically using the Pin Control library. `_WritePin(GPIOI, GPIO_PIN_8, GPIO_PIN_RESET)` is a function that writes a reset state to pin 8 on GPIOI port. This line sets the pin low or grounds it if it was previously high.
`HAL_GPIO_TogglePin(GPIOI, GPIO_PIN_8)` is used to toggle the state of pin 8 on GPIOI - meaning if it's currently high, it will be set low, and vice versa. This command performs a digital output level change.
Similarly,
`HAL_GPIO_TogglePin(GPIOC, GPIO_PIN_15)` does the same operation but for pin 15 on GPIOC port.
Here's a brief explanation:
1. `GPIOI` refers to a specific General Purpose Input/Output (GPIO) peripheral block, which is an essential part of the STM32 microcontroller's hardware.
2. `GPIO_PIN_8` and `GPIO_PIN_15` are pin numbers within the GPIOI and GPIOC peripherals respectively. They define the particular pins being manipulated.
3. `GPIO_PIN_RESET` usually represents the logical low state (0), while `GPIO_PIN_SET` would represent the logical high state (1).
To put this into action, you'd need to include the appropriate headers and initialize the GPIO before calling these functions in your main program loop.
#define IR_DO ( ( DL_GPIO_readPins( GPIO_PORT, GPIO_DO_PIN ) & GPIO_DO_PIN ) ? 1 : 0 )
`#define IR_DO ( ( DL_GPIO_readPins( GPIO_PORT, GPIO_DO_PIN ) & GPIO_DO_PIN ) ? 1 : 0 )` 这段代码是用于检查GPIO(通用输入/输出)端口上某个特定输出(DO)引脚的状态,并返回一个表示该状态的整数值。
下面是对这个表达式的详细解释:
### `DL_GPIO_readPins(GPIO_PORT, GPIO_DO_PIN)` 的作用
这里使用了宏 `DL_GPIO_readPins()` 来读取指定 GPIO 端口 (`GPIO_PORT`) 上所有引脚的状态。这个函数应该会返回一个字节(通常8位),其中每一位代表 GPIO 端口的一个引脚当前的状态,高电平(通常代表“ON”或者“1”,用逻辑真值表示)或者低电平(通常代表“OFF”或者“0”,用逻辑假值表示)。
### `& GPIO_DO_PIN`
这一步对从 `DL_GPIO_readPins()` 函数获取的结果和 `GPIO_DO_PIN` 进行按位与操作(`AND` operation)。这样做是为了仅关注我们感兴趣的 DO 引脚的状态,忽略了其他引脚的信息。
### `? 1 : 0`
最后的部分是一个三元运算符,其基本形式是 `(condition) ? value_if_true : value_if_false`。在这里:
- `condition`: 是上面按位与操作的结果。如果 `DO` 引脚处于高电平状态,则结果为非零值(例如,1 或者其他的非零二进制数);如果是低电平状态,则结果为零(0)。
- `value_if_true`: 如果条件满足(即 DO 引脚高电平),则返回 1。
- `value_if_false`: 如果条件不满足(即 DO 引脚低电平),则返回 0。
所以整个表达式 `#define IR_DO ( ( DL_GPIO_readPins( GPIO_PORT, GPIO_DO_PIN ) & GPIO_DO_PIN ) ? 1 : 0 )` 实际上是检查 DO 引脚是否处于高电平状态。如果处于高电平,IR_DO 宏的定义将是 `1`,反之则是 `0`。
### 示例应用
例如,如果你正在编写一个程序来检测是否有红外信号通过 DO 引脚传输,你可以将此表达式放在适当的位置来检查 DO 引脚的状态,然后据此做出相应的响应。
---
阅读全文
相关推荐
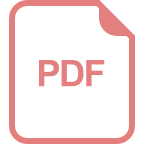
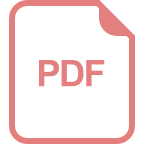
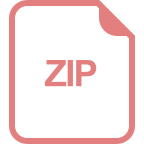













