设指针la和lb分别指向两个无头结点单链表中的首元结点,试设计从表la中删除自第i个元素起共len个元素,并将它们插入到表lb的第j个元素之后的算法。用C语言实现
时间: 2024-10-12 22:16:14 浏览: 39
在C语言中,我们可以设计如下的算法来实现这个功能。首先,我们有一个基本思路:遍历第一个链表(la),取出需要删除的部分并存储在一个临时链表中,然后从第二个链表(lb)开始找到插入位置,最后将临时链表的头节点插入到合适的位置。
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 拷贝链表的一部分到临时链表
Node* copySubList(Node* source, int len) {
Node* tail = malloc(sizeof(Node));
tail->data = source->data;
tail->next = NULL;
if (source->next) {
Node* temp = source->next;
for (int i = 1; i < len && temp; i++, temp = temp->next) {
Node* newNode = malloc(sizeof(Node));
newNode->data = temp->data;
newNode->next = tail;
tail = newNode;
}
}
return tail;
}
// 插入链表
void insertAfter(Node** target, Node* newNode, int pos) {
Node* current = *target;
int count = 0;
if (current) {
while (count < pos - 1 && current->next) {
current = current->next;
count++;
}
if (count < pos - 1) {
printf("Invalid insertion position.\n");
return;
}
newNode->next = current->next;
current->next = newNode;
}
}
// 删除链表部分并在另一链表中插入
void deleteAndInsert(Node** la, Node** lb, int i, int len, int j) {
Node* subListTail = copySubList(*la, len);
Node* lbCurrent = *lb;
// 删除la的部分
for (int k = 0; k < len; k++) {
(*la)->data = (*la)->next->data;
(*la) = (*la)->next;
}
// 如果lb为空或j <= 0,将subList插入到最前面
if (!*lb || j <= 0) {
insertAfter(lb, subListTail, 1);
} else {
// 否则按照指定位置插入
insertAfter(&lbCurrent, subListTail, j);
}
}
// 示例链表函数
Node* createList() { /* 创建链表函数 */ }
void printList(Node* head) { /* 打印链表函数 */ }
int main() {
Node* la = createList(); // 表la
Node* lb = createList(); // 表lb
int i = 1, len = 3, j = 2; // 切割位置和插入位置
printf("Before operations:\n");
printList(la);
printList(lb);
deleteAndInsert(&la, &lb, i, len, j);
printf("\nAfter operations:\n");
printList(la);
printList(lb);
return 0;
}
```
这段代码首先实现了复制链表的一部分(copySubList)、插入链表(insertAfter)以及整个删除和插入操作(deleteAndInsert)。请注意,这只是一个基础示例,实际应用中还需要考虑内存管理以及错误处理。
阅读全文
相关推荐
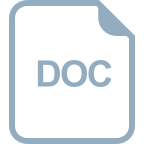
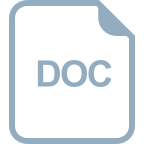
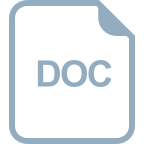















