C语言写一个串口1中断处理接收16进制数据的函数
时间: 2024-10-13 08:15:19 浏览: 25
在C语言中,编写一个串口1中断处理程序以接收16进制数据通常涉及以下几个步骤:
首先,你需要包含一些头文件,比如`stdio.h`、`stdlib.h`、`fcntl.h`、`termios.h` 和 `unistd.h`,以及相关的硬件库(如`sys/ioctl.h` 和 `asm` 根据具体的硬件平台和操作系统调整)。
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <string.h>
// 假设我们有一个结构体来表示串口配置
struct serial_config {
int fd; // 文件描述符
struct termios config;
};
// 接收16进制数据的函数
void receive_hex_data(struct serial_config *ser) {
char buffer[20]; // 假设最多能接收16位的16进制数据
ssize_t bytes_received;
while (1) { // 循环等待新数据
bytes_received = read(ser->fd, buffer, sizeof(buffer));
if (bytes_received > 0) {
buffer[bytes_received] = '\0'; // 将接收到的数据截断
// 现在需要解析这个缓冲区,将16进制字符串转换为整数
uint16_t data = strtol(buffer, NULL, 16); // 使用strtol函数
// 这里你可以进一步处理data,例如打印、存储等
printf("Received hex data: %X\n", data);
} else if (bytes_received == 0) {
// 如果读取到EOF,可能表示连接关闭
break;
} else { // 错误,可能是干扰信号或其他异常
perror("Error reading from serial port");
break;
}
}
}
```
阅读全文
相关推荐
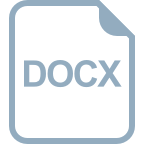
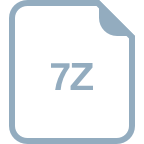















