class PolygonZone: """ A class for defining a polygon-shaped zone within a frame for detecting objects. Attributes: polygon (np.ndarray): A polygon represented by a numpy array of shape `(N, 2)`, containing the `x`, `y` coordinates of the points. frame_resolution_wh (Tuple[int, int]): The frame resolution (width, height) triggering_position (Position): The position within the bounding box that triggers the zone (default: Position.BOTTOM_CENTER) current_count (int): The current count of detected objects within the zone mask (np.ndarray): The 2D bool mask for the polygon zone """ def __init__( self, polygon: np.ndarray, frame_resolution_wh: Tuple[int, int], triggering_position: Position = Position.BOTTOM_CENTER, ): self.polygon = polygon.astype(int) self.frame_resolution_wh = frame_resolution_wh self.triggering_position = triggering_position self.current_count = 0 width, height = frame_resolution_wh self.mask = polygon_to_mask( polygon=polygon, resolution_wh=(width + 1, height + 1) )
时间: 2024-02-14 10:30:19 浏览: 579
这是一个名为 `PolygonZone` 的类,用于定义帧中的多边形区域,用于检测对象。
该类具有以下属性:
- `polygon`:一个形状为 `(N, 2)` 的numpy数组,表示由 `x` 和 `y` 坐标组成的多边形的点。
- `frame_resolution_wh`:帧的分辨率(宽度,高度)的元组。
- `triggering_position`:触发区域内的位置(默认为 Position.BOTTOM_CENTER)。
- `current_count`:区域内检测到的对象的当前计数。
- `mask`:多边形区域的二维布尔掩码。
`PolygonZone` 类有一个初始化方法 `__init__`,它接受多边形、帧分辨率和触发位置作为参数。在初始化过程中,它将多边形转换为整数类型,并根据分辨率生成一个二维掩码。
以下是代码示例:
```python
import numpy as np
from typing import Tuple
class PolygonZone:
def __init__(
self,
polygon: np.ndarray,
frame_resolution_wh: Tuple[int, int],
triggering_position: Position = Position.BOTTOM_CENTER,
):
self.polygon = polygon.astype(int)
self.frame_resolution_wh = frame_resolution_wh
self.triggering_position = triggering_position
self.current_count = 0
width, height = frame_resolution_wh
self.mask = polygon_to_mask(
polygon=polygon, resolution_wh=(width + 1, height + 1)
)
```
请注意,上述代码中的 `Position` 类没有给出,您可能需要根据您的需要定义和实现它。此外,还缺少 `polygon_to_mask` 函数的实现。您需要根据您的需求自行实现该函数,以将多边形转换为掩码。
阅读全文
相关推荐
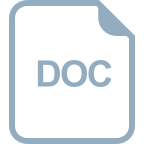
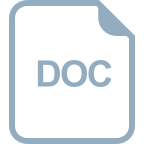
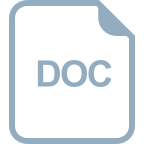
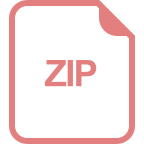












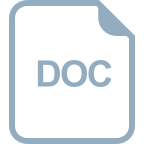
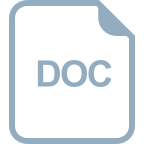