TCP通信练习3--上传文件 客户端:将本地文件上传到服务器。接收服务器的反馈。 服务器:接收客户端上传的文件,上传完毕之后给出反馈。完整代码
时间: 2024-12-08 19:18:12 浏览: 17
在Java中,使用TCP进行文件上传通常涉及到Socket编程,这里我会提供一个简单的客户端和服务器端的基本示例。请注意,这只是一个基础的例子,实际应用中可能需要考虑错误处理、文件分块传输等复杂情况。
**服务器端(Server.java):**
```java
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
private static final int PORT = 8080; // 设置监听端口
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(PORT);
System.out.println("Server started on port " + PORT);
while (true) {
Socket clientSocket = serverSocket.accept();
handleClient(clientSocket);
}
}
private static void handleClient(Socket socket) {
try (BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true)) {
String fileName = "";
System.out.print("Enter file name to upload: ");
fileName = in.readLine();
File file = new File(fileName);
if (!file.exists()) {
out.println("File not found");
} else {
FileInputStream fis = new FileInputStream(file);
byte[] buffer = new byte[(int) file.length()];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
fis.close();
out.println("File uploaded successfully");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**客户端(Client.java):**
```java
import java.io.*;
import java.net.*;
public class Client {
private static final String SERVER_ADDRESS = "localhost"; // 服务器地址
private static final int PORT = 8080;
public static void main(String[] args) throws IOException {
Socket socket = new Socket(SERVER_ADDRESS, PORT);
System.out.println("Connected to server");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintStream out = new PrintStream(socket.getOutputStream());
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the file path to be uploaded: ");
String filePath = scanner.nextLine();
File file = new File(filePath);
if (file.exists()) {
FileInputStream fis = new FileInputStream(file);
byte[] fileContent = new byte[(int) file.length()];
fis.read(fileContent);
out.write(fileContent);
out.flush();
System.out.println("Sending file...");
System.out.println("Sent file. Waiting for response...");
String response = in.readLine();
if (response.equals("File uploaded successfully")) {
System.out.println("Upload successful!");
} else {
System.out.println(response);
}
} else {
out.println("File not found");
}
}
}
```
**运行说明:**
1. 首先在服务器上运行`Server.java`。
2. 在客户端运行`Client.java`,并按照提示输入要上传的文件路径。
**相关问题--:**
1. TCP是如何支持文件上传的?
2. 上述代码中的`accept()`方法在服务器端有什么作用?
3. 客户端如何确定文件已经成功上传?
4. 如何在实际环境中防止文件过大导致传输失败?
阅读全文
相关推荐
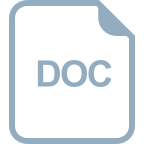
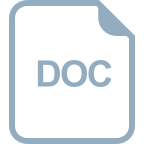
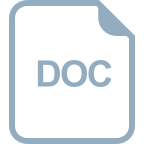


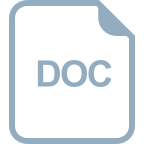
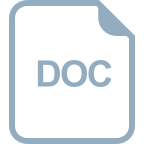
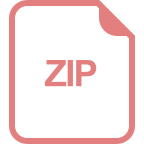
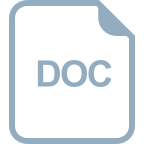
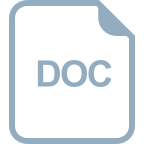
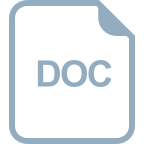
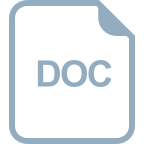
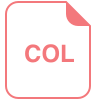




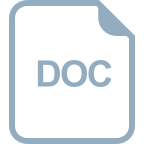
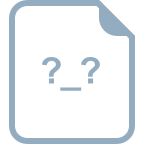