已知多个物品的重量及价格,用贪心法求背包问题的最优解。贪心法策略是优先选取单位重量价值最大的物品。的c语言代码
时间: 2024-12-02 15:27:56 浏览: 24
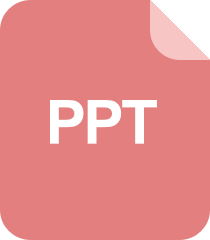
Kruskal算法-算法-贪心策略
在C语言中,可以使用贪心算法解决0/1背包问题。这是一种简单直观的方法,主要思路是在每次选择物品时,都会尽量选取单位重量价值最高的物品,直到背包装满或者所有物品都已考虑。以下是基本的贪心算法实现:
```c
#include <stdio.h>
// 定义物品结构体
typedef struct {
int weight; // 物品重量
double value; // 单位重量的价格
} Item;
// 比较两个物品单位重量的价值
int compare(const void *a, const void *b) {
Item *itemA = (Item *) a;
Item *itemB = (Item *) b;
return itemB->value / itemB->weight - itemA->value / itemA->weight;
}
// 贪心算法求解背包问题
double knapsack_greedy(Item items[], int n, int max_weight) {
qsort(items, n, sizeof(Item), compare);
double total_value = 0.0, remaining_weight = max_weight;
for (int i = 0; i < n && remaining_weight > 0; i++) {
if (items[i].weight <= remaining_weight) {
total_value += items[i].value;
remaining_weight -= items[i].weight;
} else {
total_value += items[i].value * (remaining_weight / items[i].weight);
break;
}
}
return total_value;
}
int main() {
Item items[] = { {5, 60}, {4, 100}, {6, 120} }; // 示例物品及其价格重量
int n = sizeof(items) / sizeof(items[0]);
int max_weight = 10; // 背包最大容量
printf("Using greedy algorithm, the optimal solution is worth %.2f\n", knapsack_greedy(items, n, max_weight));
return 0;
}
```
这个程序首先对物品按单位重量价值从高到低排序,然后按照贪心策略依次选择。注意,如果剩余背包空间不足以装下整个物品,会选择部分物品放入背包。
阅读全文
相关推荐
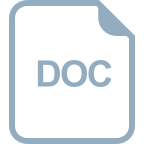
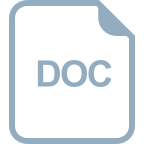

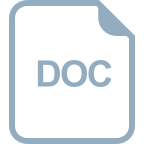


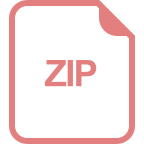
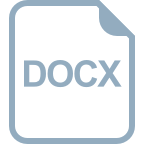
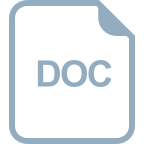
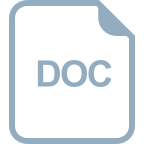
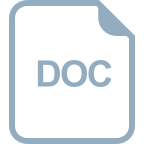
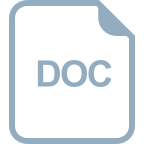
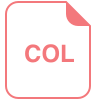
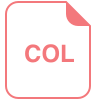
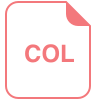


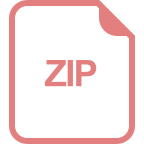