RuntimeError: The size of tensor a (16) must match the size of tensor b (15) at non-singleton dimension 1
时间: 2023-06-07 09:11:35 浏览: 288
这个错误通常是因为两个tensor在进行某些操作时维度不匹配导致的。您需要检查代码并确保您正在执行的操作是符合tensor尺寸的要求的。您还可以尝试将tensor的尺寸调整为匹配。如果您需要更多帮助,请提供更多上下文,以便我可以更好地帮助您解决这个问题。
相关问题
RuntimeError: The size of tensor a (512) must match the size of tensor b (2) at non-singleton dimension 1
遇到`RuntimeError: The size of tensor a (512) must match the size of tensor b (2) at non-singleton dimension 1`这类错误时,通常意味着两个张量(tensor a 和 tensor b)在指定维度(这里是第1维,即列数)上的大小不匹配。当你尝试执行某种操作,比如相加或矩阵乘法,如果它们的尺寸不符合运算的要求,就会抛出这个错误。
解决这个问题的方法是调整张量的尺寸以确保它们在相应维度上具有相同的大小。这通常涉及到重塑(reshape)或切片(slicing)操作。举个例子:
假设你有两个张量,a 的形状是 torch.Size([10, 512]),b 的形状是 torch.Size([10, 2]),要使它们可以相加,你需要将 b 的宽度(第二个维度)扩展到与 a 相同。你可以这样做:
```python
import torch
# 假设我们有这些张量
a = torch.randn(10, 512)
b = torch.randn(10, 2)
# 如果你想保持a的形状不变,但扩展b的宽度,可以这样操作
b_expanded = b.expand(-1, 512) # -1表示沿当前轴自动计算长度
print("扩展后的b张量形状:", b_expanded.shape)
# 然后你可以安全地做元素级相加
result = a + b_expanded
# 或者,如果你只想在特定维度上相加,可以使用 torch.gather 或 torch.index_add
# 但这取决于你的具体需求
```
RuntimeError: The size of tensor a (500) must match the size of tensor b (10) at non-singleton dimension 1
当遇到 "RuntimeError: The size of tensor a (500) must match the size of tensor b (10) at non-singleton dimension 1" 这样的错误时,这意味着两个张量在某个非单元素维度(这里是第1维)上的大小不匹配。通常,这发生在对齐运算,如矩阵乘法或卷积操作中,其中输入和输出的尺寸期望相匹配。
解决方案可能包括:
1. **检查数据预处理**:确保你在执行运算之前正确地调整了张量的形状。如果a是一个(500, *)张量,b是(10, *),你可能需要先重塑b,使其具有相同的第一个维度,如`b = b.unsqueeze(0)`,这样它就变成了`(1, 10, *)`,之后再与a进行运算。
2. **检查模型架构**:如果你在构建神经网络模型,可能是某层的输出预期的维度与实际接收到的数据不符。检查该层的输出形状设置以及是否正确地连接到了下一层。
3. **批处理处理**:如果是批量计算,确认 batch_size 是否匹配。如果你的a有500个样本,但预期的b只有10个,可能需要调整batch_size或合并小批次以达到匹配。
```python
# 示例:假设你有一个batch_size为500的输入x和期望的batch_size为10的标签y
if x.shape[0] != y.shape[0]:
if x.shape[0] > y.shape[0]:
x = x[:y.shape[0]]
else:
y = y.repeat_interleave(x.shape[0], dim=0)
```
阅读全文
相关推荐
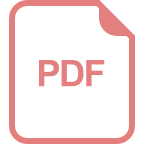
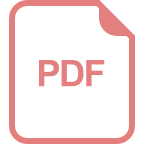
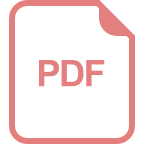












