比较两个链串的大小用C++代码语言怎么描述
时间: 2023-08-31 15:20:13 浏览: 55
### 回答1:
可以使用strcmp函数进行比较。
函数原型为:
int strcmp(const char *str1, const char *str2);
该函数返回值为:
- 若str1和str2相等,返回0;
- 若str1大于str2,返回正数;
- 若str1小于str2,返回负数。
例如,比较两个字符串s1和s2的大小,可以使用以下代码:
```c
int cmp = strcmp(s1, s2);
if (cmp == 0) {
printf("s1等于s2\n");
} else if (cmp > 0) {
printf("s1大于s2\n");
} else {
printf("s1小于s2\n");
}
```
### 回答2:
在C语言中,可以用字符串库函数strcmp()来比较两个链串的大小。字符串库函数strcmp()的原型如下:
int strcmp(const char *str1, const char *str2);
其中str1和str2是要比较的两个字符串的指针。
当str1大于str2时,strcmp()函数返回一个正数;当str1等于str2时,strcmp()返回0;当str1小于str2时,strcmp()返回一个负数。
下面是一个例子:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "abc";
char str2[] = "def";
int result = strcmp(str1, str2);
if (result > 0) {
printf("str1大于str2\n");
} else if (result < 0) {
printf("str1小于str2\n");
} else {
printf("str1等于str2\n");
}
return 0;
}
以上代码首先定义了两个要比较的链串str1和str2。然后使用strcmp()函数比较两个字符串的大小,并将结果存储在result变量中。最后根据result的值输出比较结果。
在这个例子中,输出的结果为"str1小于str2",因为字符串"abc"的ASCII码小于字符串"def"的ASCII码。
### 回答3:
比较两个链串的大小可以通过遍历链表来逐一比较两个链表中对应位置的字符的大小。可以使用C语言中的结构体来定义链表节点,节点中包含一个字符变量和指向下一个节点的指针。下面是一个描述比较链串大小的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
char data;
struct Node* next;
} Node;
// 创建节点
Node* createNode(char c) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = c;
newNode->next = NULL;
return newNode;
}
// 比较两个链串的大小
int compareStrings(Node* str1, Node* str2) {
Node* p1 = str1;
Node* p2 = str2;
while (p1 != NULL && p2 != NULL) {
if (p1->data < p2->data) {
return -1;
} else if (p1->data > p2->data) {
return 1;
}
p1 = p1->next;
p2 = p2->next;
}
if (p1 == NULL && p2 == NULL) {
return 0; // 两个链串完全相同
} else if (p1 == NULL) {
return -1; // 第一个链串是第二个链串的前缀
} else {
return 1; // 第一个链串是第二个链串的后缀
}
}
int main() {
// 创建链表1: "abcde"
Node* list1 = createNode('a');
list1->next = createNode('b');
list1->next->next = createNode('c');
list1->next->next->next = createNode('d');
list1->next->next->next->next = createNode('e');
// 创建链表2: "abc"
Node* list2 = createNode('a');
list2->next = createNode('b');
list2->next->next = createNode('c');
int result = compareStrings(list1, list2);
printf("比较结果: %d\n", result);
// 释放链表内存
Node* currentNode;
while (list1 != NULL) {
currentNode = list1;
list1 = list1->next;
free(currentNode);
}
while (list2 != NULL) {
currentNode = list2;
list2 = list2->next;
free(currentNode);
}
return 0;
}
```
在上述代码中,首先定义了一个链表节点结构体,包含一个字符变量和一个指向下一个节点的指针。然后通过`createNode`函数创建链表节点,并通过赋值操作将节点串联成一个链表。`compareStrings`函数遍历两个链串,逐一比较对应位置的字符的大小,如果遇到不相等的字符,则返回相应的结果。最后在`main`函数中创建了两个示例链表,并调用`compareStrings`函数进行比较,并打印比较结果。最后,释放链表的内存空间,避免内存泄漏。
相关推荐
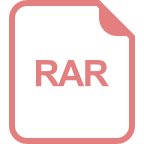
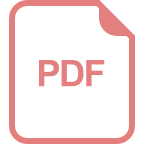














