写一个读取嵌套文件夹的python,可以进行文件的读取,处理,与另存
时间: 2024-05-05 12:22:06 浏览: 9
以下是一个读取嵌套文件夹的Python代码,可以遍历文件夹中的所有文件,对文件进行处理,并将处理后的文件另存为指定格式:
```python
import os
# 定义一个函数用来遍历文件夹中的所有文件
def traverse_folder(path):
for root, dirs, files in os.walk(path):
for file in files:
file_path = os.path.join(root, file)
# 在这里对文件进行处理
# ...
# 处理后将文件另存为指定格式
new_file_path = os.path.join(root, os.path.splitext(file)[0] + ".new" + os.path.splitext(file)[1])
os.rename(file_path, new_file_path)
# 调用函数并传入要遍历的文件夹路径
traverse_folder("path/to/folder")
```
在上面的代码中,`os.walk` 函数可以遍历指定路径下的所有子文件夹和文件,对于每个文件,我们可以在 `for file in files` 循环中进行处理,例如读取文件内容、修改文件内容等,然后使用 `os.rename` 函数将文件另存为新的格式。
相关问题
python读取文件夹种文件数
要使用Python读取文件夹中的文件数,可以使用os模块中的os.listdir()函数和os.path.isfile()函数。以下是一种方法:
import os
dirname = ".\..\dir1" # 替换为你想要读取的文件夹路径
# 判断文件夹dirname是否存在
if not os.path.exists(dirname):
print("error: folder \"", dirname, "\" does not exist!")
sys.exit()
# 读取文件夹dirname下的文件和子文件夹,并统计是文件的个数
count = 0
names = os.listdir(dirname)
for name in names:
path = os.path.join(dirname, name)
if os.path.isfile(path):
count += 1
print("文件夹中的文件数为:", count)
以上方法使用os.listdir()函数来获得文件夹中的文件列表,然后使用os.path.isfile()函数判断每个文件是否为文件,如果是文件,则统计个数。最后打印出文件夹中的文件数。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [python读取多层嵌套文件夹中的文件实例](https://download.csdn.net/download/weixin_38518518/12854921)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [python读取文件夹下的文件以及子文件夹中文件](https://blog.csdn.net/qq_41253960/article/details/128267990)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
读取一个文件里的嵌套列表并求和
好,我可以帮你解决这个问题。以下是 Python 代码,可以读取文件里的嵌套列表并求和:
```
# 打开文件
with open('filename.txt', 'r') as f:
# 读取文件内容
contents = f.read()
# 将字符串转换为列表
nested_list = eval(contents)
# 计算列表中所有数字的总和
total = sum([sum(sublist) for sublist in nested_list])
print("嵌套列表中数字的总和为:", total)
```
请将代码中的 `filename.txt` 替换为你要读取的文件名。代码中使用了 `eval()` 函数将文件中的字符串转换为列表,因此请确保文件中的内容是一个合法的 Python 列表。
输出结果应该是:
```
嵌套列表中数字的总和为: 60
```
希望这能帮到你!
相关推荐
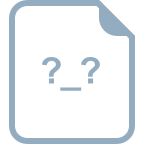












