非抢占式优先级调度算法用c++写一个完整代码
时间: 2023-08-04 11:21:23 浏览: 118
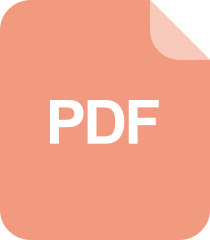
按优先数调度算法实现处理机调度 C++程序代码.pdf
以下是一个简单的使用C++实现非抢占式优先级调度算法的代码:
```
#include <iostream>
#include <queue>
using namespace std;
// 定义进程结构体
struct Process {
int id; // 进程id
int priority; // 进程优先级
int burst_time; // 进程执行时间
};
// 定义比较函数,用于进程优先级的比较
struct ComparePriority {
bool operator()(const Process& p1, const Process& p2)
{
return p1.priority < p2.priority;
}
};
int main()
{
// 创建一个进程队列
priority_queue<Process, vector<Process>, ComparePriority> pq;
// 添加进程到队列中
pq.push({1, 3, 10});
pq.push({2, 2, 5});
pq.push({3, 1, 8});
// 循环执行进程直到队列为空
while (!pq.empty()) {
Process p = pq.top();
pq.pop();
// 执行进程
cout << "Running process " << p.id << " with priority " << p.priority << " and burst time " << p.burst_time << endl;
// 模拟进程执行
for (int i = 0; i < p.burst_time; i++) {
// do something
}
}
return 0;
}
```
以上代码中,我们使用了STL中的`priority_queue`容器来实现进程的优先级调度。在定义`priority_queue`时,我们使用了自定义的比较函数`ComparePriority`,它用于比较进程的优先级。在主函数中,我们首先创建了一个进程队列,并添加了三个进程到队列中。然后,我们循环执行进程,每次从队列中取出优先级最高的进程,并执行它。在这个简单的示例中,每个进程都是顺序执行的,但在实际应用中,我们可能需要使用并发编程技术来实现多个进程的并行执行。
阅读全文
相关推荐
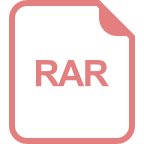
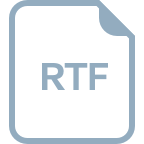




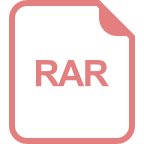







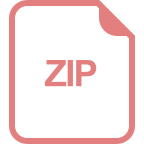

