Python用YOLOv5计算图片秧苗缺苗率代码
时间: 2023-12-25 07:13:54 浏览: 128
以下是用Python和YOLOv5计算图片中秧苗缺苗率的代码:
```python
import cv2
import torch
import numpy as np
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.torch_utils import select_device
# 加载模型
weights = 'yolov5s.pt' # 模型权重文件路径
device = select_device('') # 默认使用CUDA设备
model = attempt_load(weights, map_location=device)
img_size = 640 # 输入图像尺寸
conf_thres = 0.25 # 目标置信度阈值
iou_thres = 0.45 # NMS阈值
# 加载类别标签
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 加载输入图像
img_path = 'test.jpg' # 输入图像路径
img0 = cv2.imread(img_path) # 读取图像
img = cv2.resize(img0, (img_size, img_size)) # 缩放图像
img = img[:, :, ::-1].transpose(2, 0, 1) # BGR转RGB,HWC转CHW
img = np.ascontiguousarray(img) # 转换为连续内存
# 将图像转换为Tensor
img = torch.from_numpy(img).to(device).float()
img /= 255.0 # 转换像素值范围到0-1之间
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 模型推理
pred = model(img)[0] # 只使用第一张输出特征图
pred = non_max_suppression(pred, conf_thres, iou_thres, classes=classes)
# 绘制检测结果
for det in pred[0]:
if det is not None:
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img0.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{classes[int(cls)]} {conf:.2f}'
color = [int(c) for c in np.random.randint(0, 255, size=3)]
cv2.rectangle(img0, tuple(xyxy[:2]), tuple(xyxy[2:]), color, 2)
cv2.putText(img0, label, (xyxy[0], xyxy[1] - 10),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 显示检测结果
cv2.imshow('result', img0)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 计算缺苗率
total_count = 0 # 总秧苗数
miss_count = 0 # 缺苗秧苗数
for det in pred[0]:
if det is not None:
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img0.shape).round()
for *xyxy, conf, cls in reversed(det):
if classes[int(cls)] == 'seedling':
total_count += 1
if conf < 0.5: # 置信度小于0.5则判断为缺苗
miss_count += 1
miss_rate = miss_count / total_count * 100
print(f'Total seedling count: {total_count}')
print(f'Missed seedling count: {miss_count}')
print(f'Miss rate: {miss_rate:.2f}%')
```
请注意需要根据实际情况修改代码中的参数和文件路径。
阅读全文
相关推荐
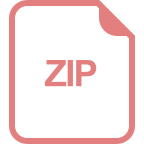
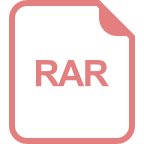





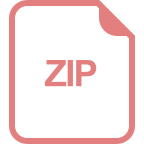
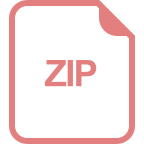
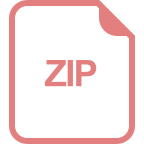
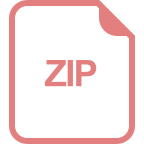
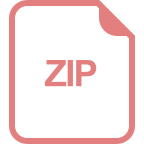
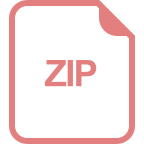
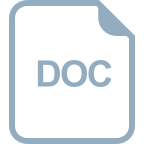
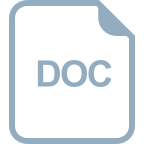
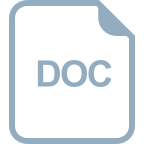
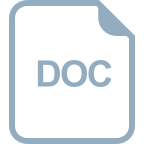
