vb.net验证码识别
时间: 2023-10-10 17:14:41 浏览: 77
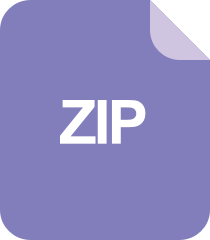
vb验证码识别

验证码识别是一项比较复杂的任务,需要使用图像处理和机器学习算法。以下是一个简单的示例,可以帮助你了解如何在 VB.NET 中识别验证码:
1. 首先,你需要从网页上获取验证码图片。可以使用 System.Net.WebClient 类来下载图片,如下所示:
```
Dim webClient As New System.Net.WebClient
webClient.DownloadFile("http://example.com/captcha.jpg", "captcha.jpg")
```
2. 接下来,你需要使用 System.Drawing.Bitmap 类加载图像文件,并将其转换为黑白图像。可以使用以下代码来实现:
```
Dim bmp As New System.Drawing.Bitmap("captcha.jpg")
For x As Integer = 0 To bmp.Width - 1
For y As Integer = 0 To bmp.Height - 1
Dim pixel As System.Drawing.Color = bmp.GetPixel(x, y)
Dim brightness As Integer = (CInt(pixel.R) + CInt(pixel.G) + CInt(pixel.B)) / 3
If brightness < 128 Then
bmp.SetPixel(x, y, System.Drawing.Color.Black)
Else
bmp.SetPixel(x, y, System.Drawing.Color.White)
End If
Next
Next
```
3. 现在,你可以使用机器学习算法来识别验证码。常用的算法包括神经网络和支持向量机(SVM)。在 VB.NET 中,可以使用 Accord.NET 框架来实现这些算法。以下是一个使用 SVM 进行验证码识别的示例:
```
Dim trainingData As New List(Of Double())
Dim trainingLabels As New List(Of Integer)
' 将训练数据和标签添加到列表中
trainingData.Add({0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}) ' digit 0
trainingLabels.Add(0)
trainingData.Add({0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}) ' digit 1
trainingLabels.Add(1)
trainingData.Add({0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}) ' digit 2
trainingLabels.Add(2)
' ...
' 创建 SVM 训练器
Dim teacher As New Accord.MachineLearning.VectorMachines.Learning.LinearSupportVectorLearning(Of Double)() With {
.Tolerance = 0.01,
.Complexity = 10000
}
Dim svm As Accord.MachineLearning.VectorMachines.SupportVectorMachine(Of Double) = teacher.Learn(trainingData.ToArray(), trainingLabels.ToArray())
' 预测验证码中每个数字的值
Dim captcha As New List(Of Integer)
For i As Integer = 0 To 3
Dim digit As New List(Of Double)
For x As Integer = i * 5 To (i + 1) * 5 - 1
For y As Integer = 0 To 9
If bmp.GetPixel(x, y).ToArgb() = System.Drawing.Color.Black.ToArgb() Then
digit.Add(1)
Else
digit.Add(0)
End If
Next
Next
captcha.Add(svm.Decide(digit.ToArray()))
Next
' 显示预测结果
MessageBox.Show(String.Join("", captcha))
```
此示例中,我们首先创建了一个包含数字 0 到 9 的训练数据集,并使用 SVM 算法训练了一个模型。然后,我们将验证码图像中的每个数字转换为一个长度为 50 的特征向量,并使用训练好的模型预测每个数字的值。最后,我们将预测结果显示在一个消息框中。
阅读全文
相关推荐

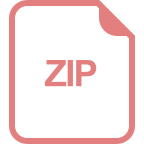
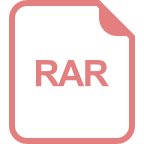
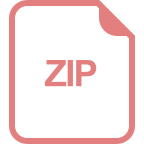
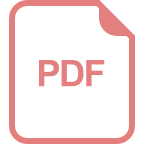
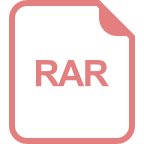
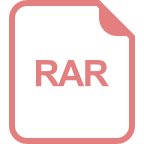
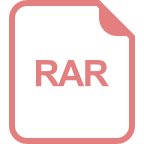
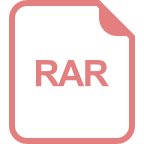
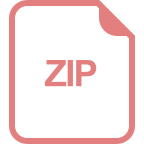
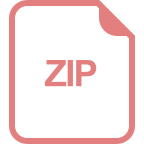
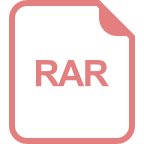
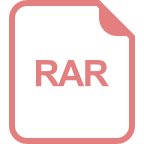
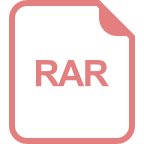
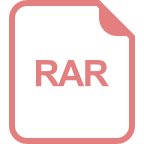