用C语言编写程序:自定义一个函数,函数的输入为一个字符串,函数的功能为统计字符串中空格符、#、!三种字符的个数,并在函数中输出三个字符的个数,函数无返回值。
时间: 2024-05-16 22:16:50 浏览: 5
下面是用 C 语言编写的程序,实现了统计字符串中空格符、#、!三种字符的个数的功能:
```c
#include <stdio.h>
void countChars(char str[]) {
int spaces = 0, hashes = 0, exclaims = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] == ' ') {
spaces++;
} else if (str[i] == '#') {
hashes++;
} else if (str[i] == '!') {
exclaims++;
}
}
printf("Number of spaces: %d\n", spaces);
printf("Number of hashes: %d\n", hashes);
printf("Number of exclamation marks: %d\n", exclaims);
}
int main() {
char str[] = "Hello #world! This is a test.";
countChars(str);
return 0;
}
```
这个程序定义了一个 `countChars` 函数,它的输入参数是一个字符串。在函数中,我们定义了三个变量来分别统计空格符、# 和!的个数。然后我们使用一个循环来遍历字符串,对于每个字符,根据它是空格符、# 还是!,我们增加相应的计数器的值。最后,我们使用 `printf` 函数来输出每个字符的个数。
在 `main` 函数中,我们定义了一个字符串 `str`,并将其作为输入参数传递给 `countChars` 函数。运行程序后,输出结果如下:
```
Number of spaces: 5
Number of hashes: 1
Number of exclamation marks: 1
```
可以看到,程序正确地统计了字符串中空格符、# 和!的个数。
相关推荐
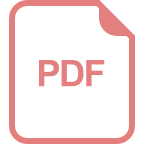














