用C语言编写一个简单程序计算后缀表达式的值(要求不多于30行)
时间: 2023-05-28 12:07:24 浏览: 98
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 30
int stack[MAX_SIZE];
int top = -1;
void push(int num)
{
if (top == MAX_SIZE - 1)
{
printf("Stack Overflow\n");
exit(1);
}
stack[++top] = num;
}
int pop()
{
if (top == -1)
{
printf("Stack Underflow\n");
exit(1);
}
return stack[top--];
}
int main()
{
char postfix[MAX_SIZE];
printf("Enter postfix expression: ");
scanf("%s", postfix);
int len = strlen(postfix);
for (int i = 0; i < len; i++)
{
if (postfix[i] >= '0' && postfix[i] <= '9')
{
push(postfix[i] - '0');
}
else
{
int num2 = pop();
int num1 = pop();
switch (postfix[i])
{
case '+':
push(num1 + num2);
break;
case '-':
push(num1 - num2);
break;
case '*':
push(num1 * num2);
break;
case '/':
push(num1 / num2);
break;
default:
printf("Invalid operator\n");
exit(1);
}
}
}
printf("Result: %d\n", pop());
return 0;
}
阅读全文
相关推荐
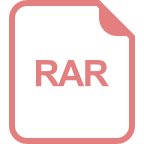
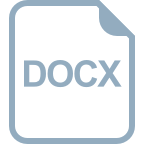
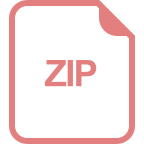
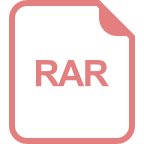
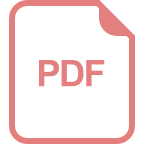
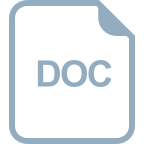
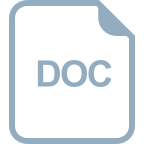
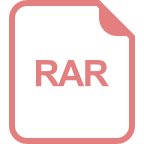
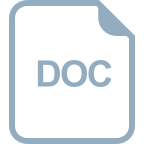
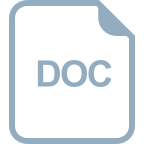
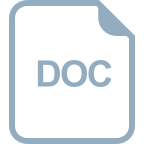
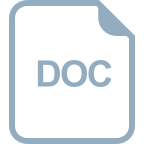
