canny算子c++
时间: 2024-12-31 21:18:22 浏览: 6
### Canny算子C++实现与使用
#### 函数定义与初始化
为了在C++中实现Canny边缘检测算法,首先需要创建必要的辅助函数来执行图像预处理操作。这包括高斯滤波器的应用以及梯度计算。
```cpp
void GaussianBlur(const Mat& inputImage, Mat& outputImage, int kernelSize, double sigma);
void SobelOperator(const Mat& srcGray, Mat& gradX, Mat& gradY, Mat& magnitude, Mat& direction);
```
这些函数用于平滑输入图片并提取其强度变化方向和大小[^1]。
#### 主要流程控制
核心部分涉及非极大值抑制(NMS),双阈值选取及边界连接等步骤:
```cpp
bool NonMaxSuppression(const Mat& mag, const Mat& dir, Mat& nms);
vector<Point> DoubleThresholdHysteresis(const Mat& imgNms, float lowThreshRatio, float highThreshRatio);
void DrawEdges(Mat& image, vector<Point>& edges);
```
此段代码实现了完整的Canny过程,从原始灰度图到最终二值化后的轮廓线绘制[^2]。
#### 完整示例程序结构
下面给出一个简化版的例子展示如何调用上述各个组件完成整个工作流:
```cpp
int main() {
// 加载测试图像
Mat originalImg = imread("path_to_image", IMREAD_GRAYSCALE);
if (originalImg.empty()) {
cout << "Could not open or find the image" << endl;
return -1;
}
Mat blurredImg, gradientMagnitude, edgeDirection, nonMaxSuppressed, cannyOutput;
// 应用Gaussian模糊减少噪声影响
GaussianBlur(originalImg, blurredImg, 5, 1.4);
// 使用Sobel算子求取梯度幅值和角度
SobelOperator(blurredImg, _, _, gradientMagnitude, edgeDirection);
// 执行非最大抑制去除伪响应点
NonMaxSuppression(gradientMagnitude, edgeDirection, nonMaxSuppressed);
// 双阈值法筛选真实边缘像素
std::vector<cv::Point> strongEdges = DoubleThresholdHysteresis(nonMaxSuppressed, 0.1f, 0.3f);
// 将找到的边画出来
DrawEdges(cannyOutput, strongEdges);
imshow("Detected Edges", cannyOutput);
waitKey();
}
```
通过这段代码可以理解Canny算子的工作机制及其具体应用方式。值得注意的是实际项目里可能还需要考虑更多细节优化性能表现。
阅读全文
相关推荐



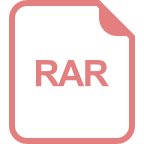

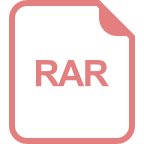











