python字典怎样用c实现
时间: 2023-12-29 13:54:21 浏览: 140
Python中的字典是一种哈希表的实现,C语言中也可以使用哈希表来实现字典。
以下是一个简单的使用C语言实现字典的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 1000
typedef struct {
char* key;
char* value;
} Entry;
typedef struct {
Entry* entries[TABLE_SIZE];
} Dictionary;
int hash(char* key) {
int hash = 0;
for (int i = 0; i < strlen(key); i++) {
hash = (hash * 31 + key[i]) % TABLE_SIZE;
}
return hash;
}
Entry* create_entry(char* key, char* value) {
Entry* entry = malloc(sizeof(Entry));
entry->key = malloc(strlen(key) + 1);
entry->value = malloc(strlen(value) + 1);
strcpy(entry->key, key);
strcpy(entry->value, value);
return entry;
}
Dictionary* create_dictionary() {
Dictionary* dictionary = malloc(sizeof(Dictionary));
memset(dictionary->entries, 0, sizeof(Entry*) * TABLE_SIZE);
return dictionary;
}
void put(Dictionary* dictionary, char* key, char* value) {
int index = hash(key);
Entry* entry = dictionary->entries[index];
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
free(entry->value);
entry->value = malloc(strlen(value) + 1);
strcpy(entry->value, value);
return;
}
entry = entry->next;
}
entry = create_entry(key, value);
entry->next = dictionary->entries[index];
dictionary->entries[index] = entry;
}
char* get(Dictionary* dictionary, char* key) {
int index = hash(key);
Entry* entry = dictionary->entries[index];
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
return entry->value;
}
entry = entry->next;
}
return NULL;
}
void delete(Dictionary* dictionary, char* key) {
int index = hash(key);
Entry* entry = dictionary->entries[index];
Entry* prev = NULL;
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
if (prev == NULL) {
dictionary->entries[index] = entry->next;
} else {
prev->next = entry->next;
}
free(entry->key);
free(entry->value);
free(entry);
return;
}
prev = entry;
entry = entry->next;
}
}
void free_dictionary(Dictionary* dictionary) {
for (int i = 0; i < TABLE_SIZE; i++) {
Entry* entry = dictionary->entries[i];
while (entry != NULL) {
Entry* next = entry->next;
free(entry->key);
free(entry->value);
free(entry);
entry = next;
}
}
free(dictionary);
}
int main() {
Dictionary* dictionary = create_dictionary();
put(dictionary, "one", "1");
put(dictionary, "two", "2");
put(dictionary, "three", "3");
printf("%s\n", get(dictionary, "one"));
printf("%s\n", get(dictionary, "two"));
printf("%s\n", get(dictionary, "three"));
delete(dictionary, "two");
printf("%s\n", get(dictionary, "two"));
free_dictionary(dictionary);
return 0;
}
```
该代码使用了一个哈希表来实现字典,其中哈希函数使用了一种简单的方式来计算字符串的哈希值,每个桶中的元素使用链表来解决哈希冲突问题。使用该代码可以实现类似Python中字典的put、get和delete操作。
阅读全文
相关推荐
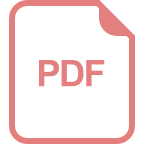
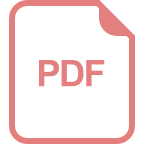













