规划问题的求解 ● 原理分析或设计(写出:任务是什么,你采用什么技术或方法完成任务,该技术的原理/公式是什么,技术实施的关键步骤有哪几个) ● 编程实现上述工作,全部代码写到下面(复制粘贴代码,添加必要的注释说明):
时间: 2024-02-22 18:58:05 浏览: 19
任务:采用A*算法求解机器人在方格地图中的路径规划问题。
采用的方法:A*算法,是一种启发式搜索算法,通过估价函数来评估当前节点的优先级,从而进行搜索。
算法原理:
1. 定义估价函数f(n):f(n) = g(n) + h(n)
- g(n):起点到当前节点的实际代价
- h(n):当前节点到终点的估计代价
2. 将起点加入open列表。
3. 从open列表中选出f(n)最小的节点n,将其从open列表中删除,加入close列表。
4. 对n的相邻节点m进行如下操作:
- 如果m已经在close列表中,则忽略它。
- 如果m不在open列表中,则将m加入open列表,并将m的父节点设置为n。计算m的f、g、h值。
- 如果m已经在open列表中,则比较n到m的g值和m当前的g值,如果n到m的g值更小,则更新m的父节点为n,并重新计算m的f、g、h值。
5. 如果终点在close列表中,则搜索结束,返回路径。
6. 如果open列表为空,则搜索失败,不存在可行路径。
关键步骤:
1. 定义状态类,包含机器人的位置和已走过的路径。
2. 实现估价函数f(n)、计算g(n)和h(n)的函数。
3. 初始化起点和终点,将起点加入open列表。
4. 实现A*算法的主循环,直到open列表为空或终点在close列表中。
5. 返回从起点到终点的路径。
下面是代码实现(Python):
``` python
class State:
def __init__(self, x, y, path):
self.x = x
self.y = y
self.path = path
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def __hash__(self):
return hash((self.x, self.y))
def distance(x1, y1, x2, y2):
return abs(x1 - x2) + abs(y1 - y2)
def f(state, goal):
return distance(state.x, state.y, goal.x, goal.y) + len(state.path)
def search(start, goal, obstacles):
open_list = [start]
close_list = set()
while open_list:
current = min(open_list, key=lambda x: f(x, goal))
open_list.remove(current)
close_list.add(current)
if current == goal:
return current.path + [goal]
for dx, dy in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
x, y = current.x + dx, current.y + dy
if 0 <= x < len(obstacles) and 0 <= y < len(obstacles[0]) and not obstacles[x][y]:
next_state = State(x, y, current.path + [current])
if next_state in close_list:
continue
if next_state not in open_list:
open_list.append(next_state)
else:
index = open_list.index(next_state)
if len(next_state.path) < len(open_list[index].path):
open_list[index] = next_state
return None
# 测试
obstacles = [[0, 0, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0]]
start = State(0, 0, [])
goal = State(4, 4, [])
path = search(start, goal, obstacles)
if path:
for state in path:
print(state.x, state.y)
else:
print("No path found.")
```
其中,obstacles表示地图障碍物的位置,0表示可以通行的位置,1表示障碍物;start和goal分别表示起点和终点的位置;path为从起点到终点的路径,如果路径不存在则为None。
相关推荐
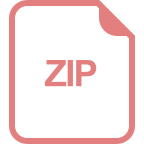
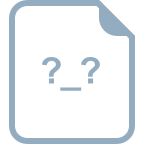














