Go语言读写锁使用案例代码
时间: 2023-04-01 16:02:43 浏览: 157
以下是一个使用 Go 语言读写锁的示例代码:
```
package main
import (
"fmt"
"sync"
)
type Counter struct {
value int
mu sync.RWMutex
}
func (c *Counter) Increment() {
c.mu.Lock()
defer c.mu.Unlock()
c.value++
}
func (c *Counter) Decrement() {
c.mu.Lock()
defer c.mu.Unlock()
c.value--
}
func (c *Counter) Value() int {
c.mu.RLock()
defer c.mu.RUnlock()
return c.value
}
func main() {
c := Counter{value: }
var wg sync.WaitGroup
for i := ; i < 100; i++ {
wg.Add(1)
go func() {
c.Increment()
wg.Done()
}()
}
wg.Wait()
fmt.Println(c.Value())
}
```
这个示例代码定义了一个 Counter 结构体,其中包含一个整数值和一个读写锁。Increment 和 Decrement 方法分别对值进行加一和减一的操作,使用写锁来保证并发安全。Value 方法用于获取当前值,使用读锁来保证并发安全。在主函数中,启动了 100 个 goroutine 来对 Counter 进行加一操作,最后输出 Counter 的值。
阅读全文
相关推荐
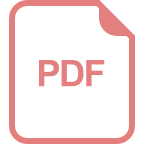
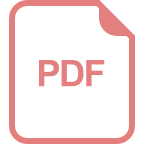
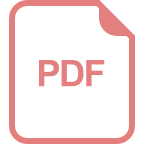














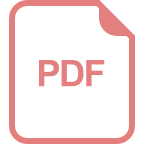
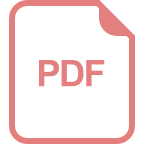