mapreduce实现两个表连接
时间: 2023-06-27 17:03:03 浏览: 147
在 MapReduce 中实现两个表的连接可以通过以下步骤:
1. 在 Map 阶段,对两个待连接的表进行拆分,生成键值对,其中键为连接的关键字,值为待连接的记录。
2. 将生成的键值对进行分组,将具有相同键的记录放在同一个组中。
3. 在 Reduce 阶段,对于每个组,进行表连接操作,将两个表中符合条件的记录连接起来,生成新的记录。
下面是一个示例代码,假设有两个表,一个是订单表 orders,包含订单编号、客户编号和订单金额等字段;另一个是客户表 customers,包含客户编号、客户姓名和联系方式等字段。现在需要按照客户编号连接这两个表,生成客户订单表,包含客户编号、客户姓名、联系方式和订单金额等字段。
Mapper 代码:
```
public class JoinMapper extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
if (fields.length == 3) { // customers 表
String customerId = fields[0];
String customerName = fields[1];
String customerContact = fields[2];
context.write(new Text(customerId), new Text("c#" + customerName + "#" + customerContact));
} else if (fields.length == 4) { // orders 表
String orderId = fields[0];
String customerId = fields[1];
String orderAmount = fields[2];
context.write(new Text(customerId), new Text("o#" + orderId + "#" + orderAmount));
}
}
}
```
Reducer 代码:
```
public class JoinReducer extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
String customerName = null;
String customerContact = null;
List<String> orderIds = new ArrayList<>();
List<String> orderAmounts = new ArrayList<>();
for (Text value : values) {
String[] fields = value.toString().split("#");
if (fields[0].equals("c")) {
customerName = fields[1];
customerContact = fields[2];
} else if (fields[0].equals("o")) {
orderIds.add(fields[1]);
orderAmounts.add(fields[2]);
}
}
if (customerName != null) {
for (int i = 0; i < orderIds.size(); i++) {
String orderId = orderIds.get(i);
String orderAmount = orderAmounts.get(i);
context.write(new Text(key + "," + customerName + "," + customerContact), new Text(orderId + "," + orderAmount));
}
}
}
}
```
以上代码中,Mapper 阶段将两个表的记录拆分成键值对,其中键为客户编号,值为客户或订单记录,并通过前缀标识来区分是客户还是订单。在 Reduce 阶段,对于每个客户编号,将其对应的所有客户和订单记录进行连接操作,生成新的客户订单记录。最终输出的结果是以客户编号为键,客户姓名、联系方式、订单编号和订单金额为值的键值对。
阅读全文
相关推荐
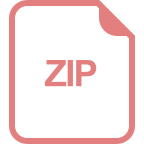
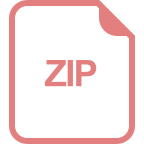
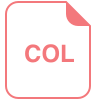




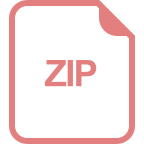
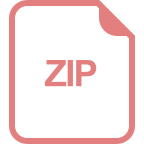
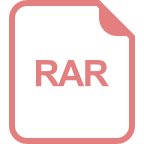
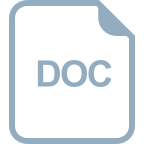
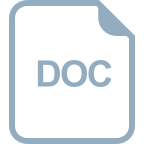
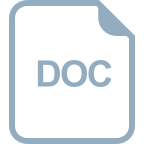
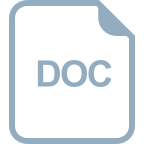
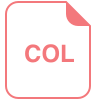
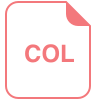

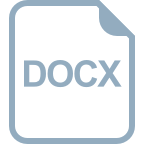