写一个加密程序,对文件1.txt进行加密。它从输入流中读入一个密钥,并将文件密文输出。采用下面的简单加密算法: 1)读入密钥的key是一个字符串; 2)明文中字符c的密文为c^key[i]; 3)循环使用key中的各个字符,直至处理完全部明文。如果key为空,则不作加密 4)解密时,用同一个key重新加密密文,就可以得到明文。 c语言代码
时间: 2024-02-15 08:02:20 浏览: 106
好的,这是一个简单的加密程序的C语言代码,可以对文件进行加密:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_KEY_LEN 100
int main(void) {
FILE *fp_in, *fp_out;
char key[MAX_KEY_LEN + 1];
int key_len, key_index = 0;
int c;
// 打开输入文件
fp_in = fopen("1.txt", "r");
if (fp_in == NULL) {
fprintf(stderr, "Error: cannot open input file\n");
return 1;
}
// 打开输出文件
fp_out = fopen("1_encrypted.txt", "w");
if (fp_out == NULL) {
fprintf(stderr, "Error: cannot open output file\n");
fclose(fp_in);
return 1;
}
// 读入密钥
printf("Please enter the encryption key: ");
scanf("%s", key);
key_len = strlen(key);
// 逐字符加密并输出密文
while ((c = fgetc(fp_in)) != EOF) {
if (key_len > 0) {
c ^= key[key_index++ % key_len];
}
fputc(c, fp_out);
}
// 关闭文件
fclose(fp_in);
fclose(fp_out);
printf("Encryption successful.\n");
return 0;
}
```
该程序首先打开输入文件 `1.txt` 和输出文件 `1_encrypted.txt`,然后读入密钥并计算密钥长度。随后,它逐字符读入输入文件中的明文,对每个字符执行简单异或加密算法,并将密文输出到输出文件中。最后,程序关闭文件并输出加密成功的消息。
阅读全文
相关推荐
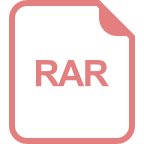



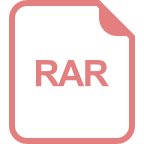
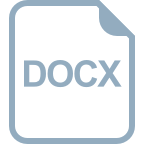
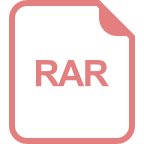

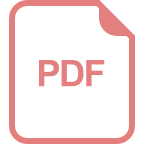
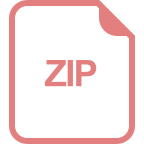
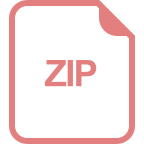
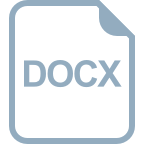