如何在c++中,贪吃蛇游戏中,vector有什么作用
时间: 2024-04-05 18:35:44 浏览: 100
在贪吃蛇游戏中,vector可以用来存储蛇身的位置,因为蛇的身体是不断增长的,而vector可以动态地扩展容量,方便地添加新的蛇身位置。同时,vector还可以用来存储食物的位置,以便于判断蛇是否吃到了食物。
在具体实现中,可以定义一个存储蛇身位置的vector,每当蛇移动时,就将蛇头的位置加入vector的末尾,同时将vector的头部元素删除,以实现蛇身的移动。当蛇吃到食物时,就将食物的位置加入vector的末尾,以实现蛇身的增长。
相关问题
怎么样在c++中使用vector创造贪吃蛇游戏的边界
使用 vector 来实现贪吃蛇游戏的边界,可以通过初始化一个二维 vector,将边界的位置标记为特殊值,然后在游戏中判断贪吃蛇是否撞到了边界。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
const int ROWS = 10; // 场景行数
const int COLS = 20; // 场景列数
const int WALL = -1; // 边界标记
vector<vector<int>> scene(ROWS, vector<int>(COLS, 0)); // 场景
void initScene()
{
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (i == 0 || i == ROWS - 1 || j == 0 || j == COLS - 1)
{
scene[i][j] = WALL; // 边界
}
}
}
}
void printScene()
{
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (scene[i][j] == WALL)
{
cout << "#"; // 边界符号
}
else
{
cout << " "; // 空白符号
}
}
cout << endl;
}
}
int main()
{
initScene(); // 初始化场景
printScene(); // 打印场景
return 0;
}
```
在上面的代码中,`initScene()` 函数用来初始化场景,将边界位置的值设置为特殊值 `WALL`,表示这些位置是边界。`printScene()` 函数用来打印场景,将边界位置输出为 `#` 符号,将其他位置输出为空白符号。
初始化完成后,就可以在游戏中判断贪吃蛇是否撞到了边界,如果撞到了边界,就可以结束游戏了。
用c++中的类实现贪吃蛇游戏
好的,以下是一个简单的贪吃蛇游戏实现。
首先,需要定义一个Snake类来保存蛇的信息,包括蛇身的坐标和蛇头的方向,以及蛇是否存活等信息。
```C++
class Snake {
public:
Snake(int init_size, int width, int height);
~Snake();
void move();
void change_direction(int direction);
bool is_alive();
void grow();
std::vector<std::pair<int, int>> get_body();
std::pair<int, int> get_head();
private:
bool check_collision();
int width_, height_;
std::deque<std::pair<int, int>> body_;
int direction_;
bool alive_;
};
```
然后,实现Snake类的构造函数和析构函数:
```C++
Snake::Snake(int init_size, int width, int height) {
width_ = width;
height_ = height;
direction_ = 0;
alive_ = true;
for (int i = 0; i < init_size; ++i) {
body_.push_front(std::make_pair(i, 0));
}
}
Snake::~Snake() {
body_.clear();
}
```
接下来,实现Snake类的主要函数:
`move()`函数用来移动蛇,每次移动时需要判断蛇是否与墙或者自己相撞,如果相撞,则蛇死亡。
```C++
void Snake::move() {
auto head = body_.front();
switch (direction_) {
case 0:
head.second -= 1;
break;
case 1:
head.first += 1;
break;
case 2:
head.second += 1;
break;
case 3:
head.first -= 1;
break;
}
if (head.first < 0 || head.first >= width_ ||
head.second < 0 || head.second >= height_ ||
std::find(body_.begin() + 1, body_.end(), head) != body_.end()) {
alive_ = false;
return;
}
body_.push_front(head);
if (head == food_) {
grow();
generate_food();
}
else {
body_.pop_back();
}
}
```
`change_direction()`函数用来改变蛇的方向。
```C++
void Snake::change_direction(int direction) {
if (direction_ % 2 != direction % 2) {
direction_ = direction;
}
}
```
`is_alive()`函数用来判断蛇是否存活。
```C++
bool Snake::is_alive() {
return alive_;
}
```
`grow()`函数用来让蛇变长。
```C++
void Snake::grow() {
auto tail = body_.back();
switch (direction_) {
case 0:
tail.second += 1;
break;
case 1:
tail.first -= 1;
break;
case 2:
tail.second -= 1;
break;
case 3:
tail.first += 1;
break;
}
body_.push_back(tail);
}
```
`get_body()`函数和`get_head()`函数用来获取蛇身和蛇头的坐标。
```C++
std::vector<std::pair<int, int>> Snake::get_body() {
std::vector<std::pair<int, int>> coordinates(body_.begin(), body_.end());
return coordinates;
}
std::pair<int, int> Snake::get_head() {
return body_.front();
}
```
最后,实现main函数来启动游戏。
```C++
int main() {
const int WIDTH = 20;
const int HEIGHT = 20;
const int INIT_SIZE = 3;
const int FPS = 10;
Snake snake(INIT_SIZE, WIDTH, HEIGHT);
sf::RenderWindow window(sf::VideoMode(WIDTH * BLOCK_SIZE, HEIGHT * BLOCK_SIZE), "Snake");
window.setFramerateLimit(FPS);
while (window.isOpen() && snake.is_alive()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed) {
window.close();
}
else if (event.type == sf::Event::KeyPressed) {
switch (event.key.code) {
case sf::Keyboard::Up:
snake.change_direction(0);
break;
case sf::Keyboard::Right:
snake.change_direction(1);
break;
case sf::Keyboard::Down:
snake.change_direction(2);
break;
case sf::Keyboard::Left:
snake.change_direction(3);
break;
}
}
}
window.clear();
snake.move();
auto body = snake.get_body();
for (auto& block : body) {
sf::RectangleShape rectangle(sf::Vector2f(BLOCK_SIZE, BLOCK_SIZE));
rectangle.setPosition(block.first * BLOCK_SIZE, block.second * BLOCK_SIZE);
rectangle.setFillColor(sf::Color::Green);
window.draw(rectangle);
}
window.display();
sf::sleep(sf::milliseconds(1000 / FPS));
}
return 0;
}
```
这里使用了SFML库来绘制游戏界面,需要在编译时链接该库。完整代码如下:
阅读全文
相关推荐
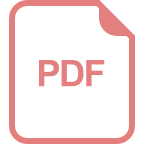
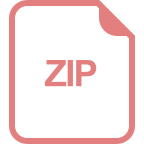
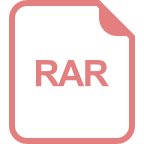







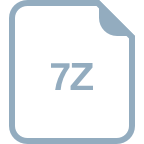
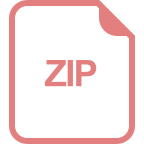
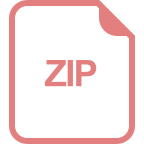
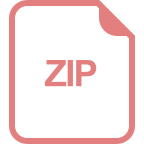
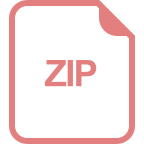
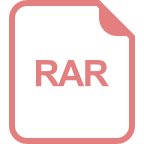
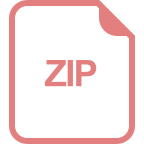