c++写一个贪吃蛇小游戏带有颜色
时间: 2024-01-06 12:04:10 浏览: 78
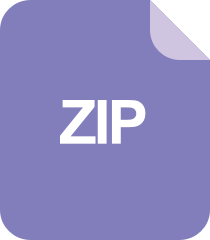
30个c++语言小游戏项目源码完美运行

以下是一个简单的 C++ 贪吃蛇游戏示例,使用了 Windows API 中的控制台颜色设置来实现颜色:
```c++
#include <iostream>
#include <conio.h>
#include <windows.h>
#include <vector>
using namespace std;
const int consoleWidth = 80; // 控制台窗口宽度
const int consoleHeight = 25; // 控制台窗口高度
const int snakeInitialLength = 3; // 贪吃蛇初始长度
// 贪吃蛇结构体,包含蛇身和方向
struct Snake {
vector<pair<int, int>> body; // 蛇身,存储每个蛇节的坐标
int direction; // 方向,0-上,1-下,2-左,3-右
};
// 游戏状态枚举,包括游戏进行中、失败和胜利
enum class GameState {
Playing,
Failed,
Win
};
// 食物结构体,包含位置
struct Food {
pair<int, int> position; // 食物位置
};
// 控制台颜色枚举,用于设置控制台输出颜色
enum ConsoleColor {
Black = 0,
Blue = 1,
Green = 2,
Cyan = 3,
Red = 4,
Magenta = 5,
Brown = 6,
LightGray = 7,
DarkGray = 8,
LightBlue = 9,
LightGreen = 10,
LightCyan = 11,
LightRed = 12,
LightMagenta = 13,
Yellow = 14,
White = 15
};
// 设置控制台输出颜色
void SetConsoleTextColor(ConsoleColor color) {
HANDLE consoleHandle = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(consoleHandle, static_cast<WORD>(color));
}
// 在控制台指定位置输出字符,坐标从左上角开始计算
void SetCursorPosition(int x, int y) {
COORD cursorPosition = { x, y };
HANDLE consoleHandle = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorPosition(consoleHandle, cursorPosition);
}
// 隐藏控制台光标
void HideConsoleCursor() {
CONSOLE_CURSOR_INFO cursorInfo;
HANDLE consoleHandle = GetStdHandle(STD_OUTPUT_HANDLE);
GetConsoleCursorInfo(consoleHandle, &cursorInfo);
cursorInfo.bVisible = false;
SetConsoleCursorInfo(consoleHandle, &cursorInfo);
}
// 生成一个随机坐标
pair<int, int> GenerateRandomPosition() {
int x = rand() % (consoleWidth - 2) + 1;
int y = rand() % (consoleHeight - 2) + 1;
return { x, y };
}
// 在控制台输出游戏界面
void RenderGameScreen(const Snake& snake, const Food& food, const GameState& gameState) {
system("cls"); // 清屏
for (int i = 0; i < consoleHeight; i++) {
for (int j = 0; j < consoleWidth; j++) {
if (i == 0 || i == consoleHeight - 1 || j == 0 || j == consoleWidth - 1) {
SetConsoleTextColor(ConsoleColor::LightGray); // 绘制边框
cout << "#";
} else if (gameState == GameState::Failed && snake.body.front().first == j && snake.body.front().second == i) {
SetConsoleTextColor(ConsoleColor::Red); // 绘制失败的蛇头
cout << "X";
} else if (gameState == GameState::Win && snake.body.front().first == j && snake.body.front().second == i) {
SetConsoleTextColor(ConsoleColor::Yellow); // 绘制胜利的蛇头
cout << "O";
} else if (food.position.first == j && food.position.second == i) {
SetConsoleTextColor(ConsoleColor::Green); // 绘制食物
cout << "F";
} else {
bool isBodyPart = false;
for (auto& bodyPart : snake.body) {
if (bodyPart.first == j && bodyPart.second == i) {
SetConsoleTextColor(ConsoleColor::White); // 绘制蛇身
cout << "o";
isBodyPart = true;
break;
}
}
if (!isBodyPart) {
cout << " ";
}
}
}
cout << endl;
}
}
// 更新蛇的位置和状态
void UpdateSnake(Snake& snake, const Food& food, GameState& gameState) {
auto head = snake.body.front();
// 根据方向更新蛇头位置
switch (snake.direction) {
case 0: // 上
head.second--;
break;
case 1: // 下
head.second++;
break;
case 2: // 左
head.first--;
break;
case 3: // 右
head.first++;
break;
}
// 检查蛇头是否碰到边界或蛇身
if (head.first == 0 || head.first == consoleWidth - 1 || head.second == 0 || head.second == consoleHeight - 1) {
gameState = GameState::Failed; // 碰到边界,游戏失败
return;
}
for (int i = 1; i < snake.body.size(); i++) {
if (head.first == snake.body[i].first && head.second == snake.body[i].second) {
gameState = GameState::Failed; // 碰到蛇身,游戏失败
return;
}
}
snake.body.insert(snake.body.begin(), head); // 将蛇头插入蛇身
if (head.first == food.position.first && head.second == food.position.second) {
// 吃到食物
if (snake.body.size() == consoleWidth * consoleHeight - 2) {
gameState = GameState::Win; // 蛇身占满整个游戏区域,游戏胜利
return;
}
food.position = GenerateRandomPosition(); // 生成新的食物
} else {
snake.body.pop_back(); // 没有吃到食物,则删除蛇尾
}
}
// 获取用户输入的方向
void GetUserInput(Snake& snake) {
if (_kbhit()) {
int key = _getch();
switch (key) {
case 'w':
case 'W':
case 72: // 上
if (snake.direction != 1) {
snake.direction = 0;
}
break;
case 's':
case 'S':
case 80: // 下
if (snake.direction != 0) {
snake.direction = 1;
}
break;
case 'a':
case 'A':
case 75: // 左
if (snake.direction != 3) {
snake.direction = 2;
}
break;
case 'd':
case 'D':
case 77: // 右
if (snake.direction != 2) {
snake.direction = 3;
}
break;
}
}
}
int main() {
HideConsoleCursor(); // 隐藏控制台光标
srand(static_cast<unsigned int>(time(nullptr))); // 初始化随机数生成器
Snake snake;
snake.direction = 3; // 初始方向为右
int x = consoleWidth / 2;
int y = consoleHeight / 2;
for (int i = 0; i < snakeInitialLength; i++) {
snake.body.push_back({ x - i, y }); // 初始蛇身为一条水平线
}
Food food;
food.position = GenerateRandomPosition(); // 随机生成初始食物位置
GameState gameState = GameState::Playing;
while (gameState == GameState::Playing) {
RenderGameScreen(snake, food, gameState);
UpdateSnake(snake, food, gameState);
GetUserInput(snake);
Sleep(100);
}
RenderGameScreen(snake, food, gameState); // 最后一次绘制游戏界面
SetCursorPosition(consoleWidth / 2 - 4, consoleHeight / 2);
if (gameState == GameState::Failed) {
SetConsoleTextColor(ConsoleColor::Red);
cout << "Failed";
} else {
SetConsoleTextColor(ConsoleColor::Yellow);
cout << "Win";
}
SetConsoleTextColor(ConsoleColor::White);
cout << endl;
return 0;
}
```
注意:这个示例中使用了 Windows API 中的一些函数,只能在 Windows 平台上编译运行。如果您需要在其他平台上运行,请使用相应的 API 替代函数或使用跨平台库(如 SDL)。
阅读全文
相关推荐
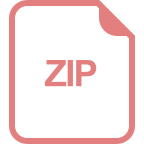
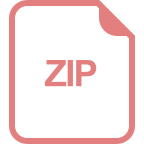










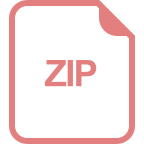
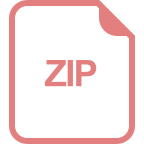
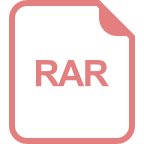
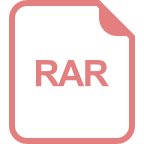
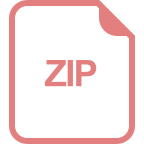
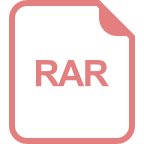