用Java多线程编程解决进程间同步和互斥问题
时间: 2024-03-17 11:46:54 浏览: 96
在Java中,可以使用synchronized关键字和wait()、notify()、notifyAll()方法来解决多线程之间的同步和互斥问题。具体实现步骤如下:
1. 定义一个共享资源类,其中包含需要互斥访问的方法。
2. 在需要互斥访问的方法前加上synchronized关键字,表示该方法是同步方法。
3. 在访问共享资源时,使用wait()方法将当前线程挂起,直到其他线程执行notify()或notifyAll()方法唤醒它。
4. 在访问共享资源完毕后,使用notify()或notifyAll()方法唤醒其他线程。
下面是一个简单的例子,实现两个线程交替打印奇偶数:
```java
class SharedResource {
private int number = 1;
private boolean isOdd = true;
public synchronized void printOdd() {
while (!isOdd) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(number);
number++;
isOdd = false;
notify();
}
public synchronized void printEven() {
while (isOdd) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(number);
number++;
isOdd = true;
notify();
}
}
public class Main {
public static void main(String[] args) {
SharedResource sharedResource = new SharedResource();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
sharedResource.printOdd();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
sharedResource.printEven();
}
});
thread1.start();
thread2.start();
}
}
```
在此例子中,首先定义了一个共享资源类SharedResource,其中包含了两个同步方法printOdd()和printEven(),分别用于打印奇数和偶数。
在printOdd()方法中,首先使用while循环判断是否可以打印奇数,如果不能,则使用wait()方法将当前线程挂起,等待其他线程唤醒它。如果可以打印奇数,则打印并将number加1,然后将isOdd设置为false表示下一个应该打印偶数,并使用notify()方法唤醒其他线程。
在printEven()方法中同理,只不过判断条件和操作的变量不同。
最后,在主函数中创建两个线程,分别调用printOdd()和printEven()方法,实现了奇偶数的交替打印。
阅读全文
相关推荐
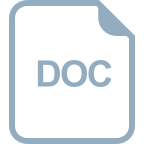
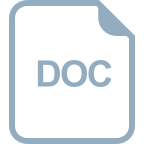
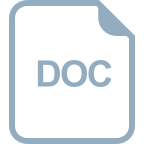





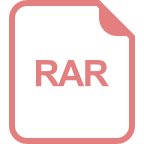
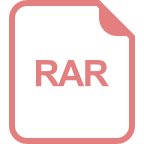
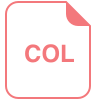
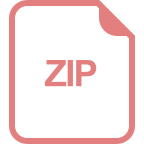
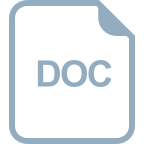
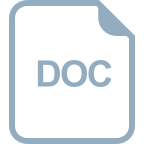
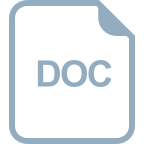
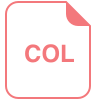
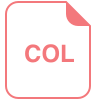
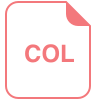
