具体代码C语言数据结构、依据键盘接收的数据,创建无向图及邻接矩阵,并输出 2、依据键盘接收的数据,创建无向图及邻接表,并输出。 3、将己知的邻接矩阵转化成邻接表,并输出。 4、读文件构造无向图,输出图的顶点数及邻接矩阵
时间: 2024-11-25 09:21:48 浏览: 71
在C语言中,我们可以使用数组或动态分配的结构体来表示无向图的数据结构。这里我将分别介绍如何通过键盘输入创建无向图的邻接矩阵、邻接表,并展示相应的操作。
**1. 邻接矩阵:**
```c
#include <stdio.h>
#define MAX_VERTICES 10
typedef struct {
int adj[MAX_VERTICES][MAX_VERTICES]; // 邻接矩阵
} Graph;
void input_graph(Graph* graph) {
int u, v;
for (int i = 0; i < MAX_VERTICES; ++i)
for (int j = 0; j < MAX_VERTICES; ++j)
graph->adj[i][j] = 0; // 初始化为非连接
printf("请输入顶点之间的关系(u v):\n");
while (scanf("%d %d", &u, &v)) { // 输入边
if (u >= 0 && u < MAX_VERTICES && v >= 0 && v < MAX_VERTICES) {
graph->adj[u][v] = 1; // 标记连接
graph->adj[v][u] = 1; // 图是无向的,所以双向
} else {
printf("非法输入!\n");
}
}
}
void print_matrix(const Graph& graph) {
for (int i = 0; i < MAX_VERTICES; ++i) {
for (int j = 0; j < MAX_VERTICES; ++j) {
printf("%d ", graph.adj[i][j]);
}
printf("\n");
}
}
// 示例
int main() {
Graph g;
input_graph(&g);
print_matrix(g);
return 0;
}
```
**2. 邻接表:**
邻接表通常用链表存储,每个节点代表一个顶点,包含一个指向邻接顶点的指针列表。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int vertex;
struct Node* next;
} Node;
typedef struct GraphAdjList {
Node* vertices[MAX_VERTICES];
} GraphAdjList;
void add_edge(GraphAdjList* graph, int src, int dest) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = dest;
newNode->next = graph->vertices[src];
graph->vertices[src] = newNode;
}
void print_adj_list(const GraphAdjList* graph) {
for (int i = 0; i < MAX_VERTICES; ++i) {
printf("顶点 %d 的邻居: ", i);
if (graph->vertices[i]) {
Node* temp = graph->vertices[i];
while (temp != NULL) {
printf("%d ", temp->vertex);
temp = temp->next;
}
} else {
printf("None\n");
}
}
}
// 示例
int main() {
GraphAdjList g;
// ... 输入和添加边到邻接表 ...
print_adj_list(&g);
return 0;
}
```
**3. 邻接矩阵转邻接表:**
这需要遍历矩阵,根据非零元素构建邻接表。
```c
void matrix_to_adj_list(GraphAdjList* adj_list, const Graph* matrix) {
for (int i = 0; i < MAX_VERTICES; ++i) {
for (int j = 0; j < MAX_VERTICES; ++j) {
if (matrix->adj[i][j]) {
add_edge(adj_list, i, j);
}
}
}
}
// 示例
void process_matrix(Graph AdjMatrix) {
GraphAdjList adjList;
matrix_to_adj_list(&adjList, &AdjMatrix);
print_adj_list(&adjList);
}
```
**4. 文件读取无向图:**
```c
#include <stdio.h>
void read_graph_from_file(char filename[], GraphAdjList* adj_list) {
FILE* file = fopen(filename, "r");
if (!file) {
printf("无法打开文件!\n");
return;
}
int vertices, edge;
fscanf(file, "%d %d", &vertices, &edge);
for (int i = 0; i < edge; ++i) {
int src, dest;
fscanf(file, "%d %d", &src, &dest);
add_edge(adj_list, src - 1, dest - 1); // 减一处理文件索引从0开始
}
fclose(file);
}
// 示例
int main() {
char filename[] = "input.txt";
GraphAdjList g;
read_graph_from_file(filename, &g);
// 输出顶点数和邻接矩阵(如果要计算并输出的话)
}
```
相关问题:
1. 邻接矩阵和邻接表各有什么优缺点?
2. 怎样在C++中更高效地实现邻接矩阵到邻接表的转换?
3. 如果文件中存储的是有向图,上述代码应该如何修改?
阅读全文
相关推荐
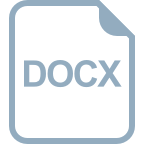
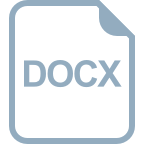
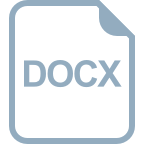















