通过vue2来实现自定义分页组件,请给出详细代码
时间: 2023-05-24 13:05:08 浏览: 63
以下是一个简单的自定义分页组件的示例,使用Vue2实现:
```
<template>
<div>
<ul class="pagination">
<li :class="{ 'disabled': currentPage === 1 }">
<a href="#" @click.prevent="prevPage">
Previous
</a>
</li>
<li v-for="page in pages" :class="{ 'active': page === currentPage }">
<a href="#" @click.prevent="changePage(page)">{{ page }}</a>
</li>
<li :class="{ 'disabled': currentPage === totalPages }">
<a href="#" @click.prevent="nextPage">
Next
</a>
</li>
</ul>
</div>
</template>
<script>
export default {
props: {
currentPage: {
type: Number,
required: true,
},
totalPages: {
type: Number,
required: true,
},
maxPagesToShow: {
type: Number,
default: 5,
},
},
computed: {
pages() {
let pages = [];
let startPage = 1;
let endPage = this.totalPages;
if (this.maxPagesToShow < this.totalPages) {
let middle = Math.ceil(this.maxPagesToShow / 2);
startPage = Math.max(this.currentPage - middle + 1, 1);
endPage = startPage + this.maxPagesToShow - 1;
if (endPage > this.totalPages) {
endPage = this.totalPages;
startPage = endPage - this.maxPagesToShow + 1;
}
}
for (let i = startPage; i <= endPage; i++) {
pages.push(i);
}
return pages;
},
},
methods: {
changePage(page) {
this.$emit('change-page', page);
},
prevPage() {
if (this.currentPage > 1) {
this.changePage(this.currentPage - 1);
}
},
nextPage() {
if (this.currentPage < this.totalPages) {
this.changePage(this.currentPage + 1);
}
},
},
};
</script>
<style>
.pagination {
display: inline-block;
}
.pagination li {
display: inline;
padding: 5px 10px;
}
.pagination li.disabled a,
.pagination li.active a {
pointer-events: none;
color: #777;
cursor: default;
}
</style>
```
在父组件中引入自定义分页组件并使用,示例代码如下:
```
<template>
<div>
<table>
<!-- 表格内容省略 -->
</table>
<custom-pagination
:current-page="currentPage"
:total-pages="totalPages"
@change-page="handlePageChange"
/>
</div>
</template>
<script>
import CustomPagination from './CustomPagination.vue';
export default {
components: {
CustomPagination,
},
data() {
return {
currentPage: 1,
totalPages: 10,
};
},
methods: {
handlePageChange(page) {
this.currentPage = page;
// 切换页面数据的方法
},
},
};
</script>
```
相关推荐
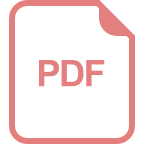
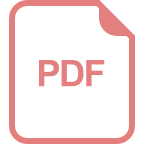
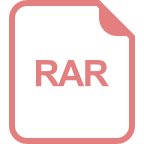
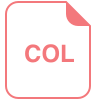













