1.编写程序求日收益,并存入变量 2.编写程序分别求每支股票的日收益变量从2015年1月6日到2021年7月7日的最大子数组。将结果保存到变量 3.利用matplotlib绘制每只股票原始价格的图线(注意:不是日收益曲线,只需画原始数据曲线)
时间: 2024-09-19 21:11:33 浏览: 39
在Python中,你可以通过以下步骤来实现这三个需求:
1. **求日收益并存入变量**:
假设我们有一个包含每日股票价格的数据列表,我们可以计算每个交易日的收盘价与前一天收盘价之间的百分比变化作为日收益率。假设数据存储在一个名为`stock_prices`的列表中:
```python
def calculate_daily_return(prices):
daily_returns = [prices[i] / prices[i - 1] - 1 for i in range(1, len(prices))]
return daily_returns
# 示例股票价格数据
stock_prices = ... # 股票每天的价格列表
daily_returns = calculate_daily_return(stock_prices)
```
2. **求最大子数组的收益**:
这通常涉及到计算子数组的累计和,并找出使其达到最大值的那个子数组。可以使用动态规划的方法实现,例如Kedro算法:
```python
from scipy.signal import argrelextrema
def max_subarray(daily_returns):
max_profit = 0
start_index = end_index = 0
current_sum = 0
for i in range(len(daily_returns)):
current_sum += daily_returns[i]
if current_sum > max_profit:
max_profit = current_sum
start_index = i - max_profit <= 0 else i
end_index = i
return daily_returns[start_index:end_index + 1]
max_subarray_result = max_subarray(daily_returns)
```
3. **绘制原始价格曲线**:
使用matplotlib库,先对原始价格数据进行排序,然后绘制折线图:
```python
import matplotlib.pyplot as plt
sorted_prices = sorted(stock_prices)
plt.plot(sorted_prices)
plt.xlabel('日期')
plt.ylabel('股票价格')
plt.title('股票原始价格曲线')
plt.show()
```
请注意,你需要提供实际的股票价格数据才能运行上述代码。另外,由于实际数据量大或复杂,这里省略了填充数据的具体部分。如果你需要更详细的帮助,请提供具体的股票价格数据结构。
阅读全文
相关推荐
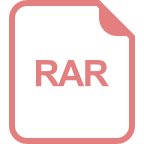
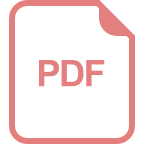
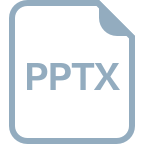





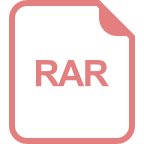
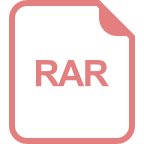
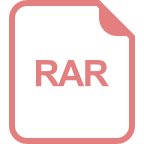
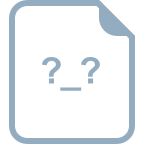
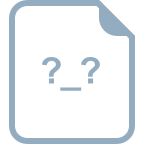
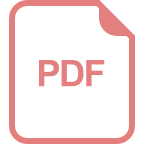
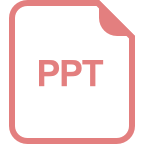
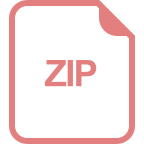
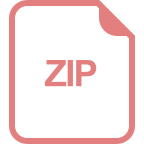