用c加加写出查找程序算法设计如果找到返回相应的下标否则返回负一假设原数组升序的代码
时间: 2024-09-20 12:12:29 浏览: 39
在C++中,你可以使用二分查找法(Binary Search Algorithm)来实现在已排序数组中查找特定元素并返回其索引的功能。以下是使用C++编写的一个简单示例:
```cpp
#include <iostream>
using namespace std;
int binarySearch(int arr[], int low, int high, int target) {
if (high >= low) {
// 找到中间元素的索引
int mid = low + (high - low) / 2;
// 检查中间元素是否是我们正在寻找的目标
if (arr[mid] == target)
return mid; // 如果找到,返回其索引
// 如果目标小于中间元素,搜索左半部分
else if (arr[mid] > target)
return binarySearch(arr, low, mid - 1, target);
// 否则,在右半部分继续搜索
else
return binarySearch(arr, mid + 1, high, target);
}
// 当目标不存在于数组中,返回-1
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9}; // 示例数组,升序排列
int n = sizeof(arr) / sizeof(arr[0]);
int target = 5; // 要查找的元素
int result = binarySearch(arr, 0, n - 1, target);
if (result != -1)
cout << "元素 " << target << " 的下标是: " << result << endl;
else
cout << "元素 " << target << " 不在数组中" << endl;
return 0;
}
```
在这个例子中,`binarySearch`函数递归地将数组分为两部分,直到找到目标值或确定目标值不在数组中为止。如果没有找到,它会返回-1。
阅读全文
相关推荐
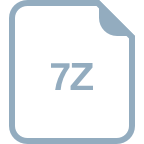
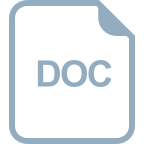
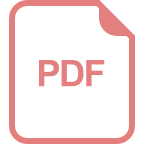















