c加加对于给定的无序数组实现升序排序,再查找大于等于给定值的位置
时间: 2024-10-27 08:13:35 浏览: 4
在 C++ 中,你可以使用 `std::sort` 函数来自头文件 `<algorithm>` 对无序数组进行升序排序,然后再遍历数组找到大于等于给定值的位置。下面是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
int find_position(const std::vector<int>& arr, int value) {
// 先对数组进行排序
std::sort(arr.begin(), arr.end());
// 使用二分查找法寻找大于等于value的位置
int pos = -1; // 初始化位置为-1表示未找到
int low = 0;
int high = arr.size() - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] >= value) {
pos = mid;
high = mid - 1; // 已经找到大于等于value的元素,缩小右边界
} else {
low = mid + 1; // 找到的第一个小于此值的元素,左边界扩大
}
}
return pos; // 返回大于等于value的第一个元素的索引
}
int main() {
std::vector<int> arr = {5, 2, 9, 1, 7};
int target = 6;
int position = find_position(arr, target);
if (position != -1) {
std::cout << "The first element greater than or equal to " << target << " is at index " << position << std::endl;
} else {
std::cout << "No element in the array is greater than or equal to " << target << std::endl;
}
return 0;
}
```
在这个例子中,`find_position` 函数首先将数组排序,然后通过二分查找找到第一个大于或等于目标值的元素的位置。如果找不到这样的元素,则返回 `-1` 表示不存在。
阅读全文
相关推荐
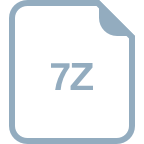
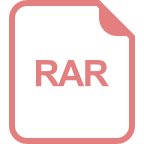
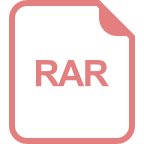















