数据结构邻接表C语言实现
时间: 2023-07-21 13:06:51 浏览: 63
邻接表是一种表示图的数据结构,可以用来存储图的顶点和边。在C语言中,可以使用链表来实现邻接表。
首先,我们需要定义图的顶点结构体和边结构体:
```c
// 边的结构体
typedef struct Edge {
int dest; // 边的目标顶点
struct Edge* next; // 指向下一条边的指针
} Edge;
// 顶点的结构体
typedef struct Vertex {
int value; // 顶点的值
Edge* head; // 指向第一条边的指针
} Vertex;
```
然后,我们可以定义邻接表的结构体,其中包含顶点数组和顶点数量:
```c
typedef struct Graph {
int numVertices; // 顶点数量
Vertex* vertices; // 顶点数组
} Graph;
```
接下来,我们可以实现邻接表的创建函数、添加边的函数和打印函数:
```c
// 创建图的邻接表
Graph* createGraph(int numVertices) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->numVertices = numVertices;
graph->vertices = (Vertex*)malloc(numVertices * sizeof(Vertex));
// 初始化顶点数组
for (int i = 0; i < numVertices; i++) {
graph->vertices[i].value = i;
graph->vertices[i].head = NULL;
}
return graph;
}
// 添加边到图的邻接表
void addEdge(Graph* graph, int src, int dest) {
Edge* newEdge = (Edge*)malloc(sizeof(Edge));
newEdge->dest = dest;
newEdge->next = graph->vertices[src].head;
graph->vertices[src].head = newEdge;
// 无向图需添加反向边
newEdge = (Edge*)malloc(sizeof(Edge));
newEdge->dest = src;
newEdge->next = graph->vertices[dest].head;
graph->vertices[dest].head = newEdge;
}
// 打印图的邻接表
void printGraph(Graph* graph) {
for (int i = 0; i < graph->numVertices; i++) {
printf("顶点 %d 的边:", i);
Edge* curr = graph->vertices[i].head;
while (curr != NULL) {
printf(" -> %d", curr->dest);
curr = curr->next;
}
printf("\n");
}
}
```
现在,我们可以在主函数中使用这些函数来创建图、添加边并打印邻接表:
```c
int main() {
int numVertices = 5;
Graph* graph = createGraph(numVertices);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
return 0;
}
```
运行以上代码,将输出如下结果:
```
顶点 0 的边: -> 4 -> 1
顶点 1 的边: -> 4 -> 3 -> 2 -> 0
顶点 2 的边: -> 3 -> 1
顶点 3 的边: -> 4 -> 2 -> 1
顶点 4 的边: -> 3 -> 1 -> 0
```
这样,我们就完成了邻接表的C语言实现。希望对你有所帮助!
相关推荐
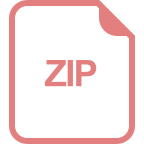
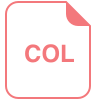
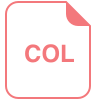
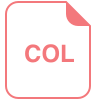
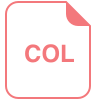
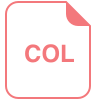









